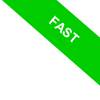
Array structures in Matlab
Now, suppose you want to learn how to use structures in Matlab. Well, let me tell you something, it's not that complicated. You see, a structure is simply a collection of data that can be accessed by name. And in Matlab, you create a structure using the "struct" function. So, let me walk you through the process of using structures in Matlab.
What are structures? Well, let me tell you. A structure is kinda like an array, but it's more powerful. See, unlike an array, a structure can store data of different types and organize them into fields. And each field of the structure has a unique name. Take a phonebook, for example. That's an example of an array structure.
How to create an array structure?
Create a structure named "phonebook".
The first field of the structure is the "name" of the contacts in the phonebook.
>> phonebook.name="John Smith";
Now add the second field of the structure.
It is the "phone number" of each contact.
>> phonebook.phone_number=123456789;
A field can contain numeric values, strings and even an array.
>> phonebook(1).phone_number=[123456789, 987654321];
Finally, add the third field of the structure to store the person's address.
>> phonebook.address="New York";
Alternatively, you can create a structure by typing all the fields in a single command using the struct() function.
>> phonebook = struct('name','John Smith','phone_number',123456789, 'address', 'New York')
You have created a structure called "phonebook" that contains one record and three fields (name, phone number, address).
Note. The fields of the structure have a different data type. Two fields contain alphanumeric values (name and address) while one field contains numeric values (phone number).
You can also create a new structure by inserting multiple records into it.
Write the field names only once and enclose the data of each individual field in curly braces.
C = struct('subject',{'Math', 'Latin', 'Science'},'grade',{27,30,18});
How to view the fields of the structure?
To display the list of fields of a structure, use the fieldnames() function.
>> fieldnames(phonebook)
ans =
{
[1,1] = name
[2,1] = phone_number
[3,1] = address
}
This function lists the fields of the structure in parentheses.
In this case, the structure has three fields: name, phone number, and address.
All the records in the structure have the same number (3) of fields and the same field names (name, phone number, and address).
How to add more data to the structure?
Once you have created the structure of fields, you can add the records to the structure, i.e. the information it will contain.
For example, to add a second record, type the name of the structure with index 2 in parentheses and the field on the left side.
Then type the value on the right side.
>> phonebook(2).name="Emily Johnson";
>> phonebook(2).phone_number="987654321";
Now the structure has two records.
When you insert a new record in the structure, you can also leave some fields empty.
For example, the address is missing in the second record of the structure.
>> phonebook(2)
ans =
scalar structure containing the fields:
name = Emily Johnson
phone_number = 987654321
address = [](0x0)
Alternatively, you can insert a new record in the structure using the struct() function.
>> phonebook(3) = struct('name','Michael Davis','phone_number',123456789, 'address', 'Los Angeles')
How to read data from a structure?
To access data within a structure, write the name of the structure with the index in parentheses. Then, add the name of the field you're interested in after a period.
For example, type phonebook(1).phone_number to read the phone number in the first record of the structure.
>> phonebook(1).phone_number
ans = 123456789
Type phonebook(2).phone_number to read the phone number in the second record.
>> phonebook(2).phone_number
ans = 987654321
Type phonebook.phone_number to display all the data in the "phone_number" field of the "phonebook" structure.
>> phonebook.phone_number
ans = 123456789
ans = 987654321
Type phonebook(1) to get all the data in the fields of the first record of the structure.
>> phonebook(1)
ans =
scalar structure containing the fields:
name = John Smith
telephone = 123456789 987654321
address = New York
How to delete a field from the structure?
To remove a field from the structure, use the function rmfield()
For example, add a new field "test" to the structure.
>> phonebook(2).test="ABC";
The structure now has four fields (name, telephone, address, test).
>> fieldnames(phonebook)
ans =
{
[1,1] = name
[2,1] = phone_number
[3,1] = address
[4,1] = test
}
Now, type rmfield(phonebook, "test") to remove the "test" field.
>> phonebook=rmfield(phonebook, "test");
The "test" field has been removed from the structure.
>> fieldnames(phonebook)
ans =
{
[1,1] = name
[2,1] = phone_number
[3,1] = address
}