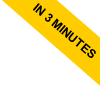
Generate random numbers from probability distributions in Octave
In this lesson I will explain how to generate a random number on Octave using different probability distributions.
Octave allows you to generate random numbers from different probability distributions (exponential, normal, poisson, gamma). Depending on the case, you can choose the most realistic one to simulate a phenomenon.
Let me give you some practical examples.
When generating a pseudorandom number with the rand() or randi() command, Octave uses the Mersenne Twister algorithm
>> rand(1)
In this case the values are uniformly distributed over all possible values.
For example, type x=rand(100) to generate a random 100x100 matrix and assign the matrix to the variable x
This command generates 10,000 random values.
>> x=rand(100);
Now display the distribution of values in a histogram using hist(x) command.
>> hist(x)
The values of the distribution are equally distributed between 0 and 1
Note. In the diagram, the horizontal axis indicates the random values from 0 to 1. The vertical axis indicates how often the values repeat.
To generate random numbers with other probability distributions you need to use different functions.
The exponential distribution
To generate a random number via exponential distribution use the function rande()
>> rande(1)
ans = 0.90844
For example, type rande(3,2) to generate a 3x2 matrix with random values
>> rande(3,2)
ans =
1.09340 0.28265
0.10781 1.72641
0.20653 0.87235
What is the difference from the uniform distribution?
To understand the difference you need to generate a random array with a larger amount of data.
For example, type x=rande(100); to generate a 100x100 array of random values.
>> x=rande(100);
Then display the frequency histogram using the hist() function
>> hist(x)
In an exponential distribution, the random values are concentrated in the lower values of the range.
Values near zero are more frequent. The frequency decreases exponentially for higher values.
The normal distribution
To generate a random number with a normal distribution use the function randn()
>> randn(1)
ans = 0.85251
Type randn(2,3) if you want to create a 2x3 matrix with random values.
>> randn(2,3)
ans =
-0.32674 2.24641 -0.19528
-0.37856 0.13721 0.41417
To understand the characteristics of the normal distribution, generate a 100x100 matrix of random values.
Type randn(100)
>> x=randn(100);
Now display the frequency histogram with hist() function
>> hist(x)
In this case, the most frequent values are those around zero.
The frequency decreases as you move away from zero to the right and left.
The normal distribution has a classic bell-shaped form.
The Poisson distribution
To generate the random numbers with the Poisson distribution use the function randp()
>> randp(1)
ans = 3
Poisson distribution generates random numbers around an average value.
Example. If the argument of the randp() function is equal to 1, the function randp(1) generates random numbers in a Poisson distribution with a mean equal to 1.
Type randp(5,2,3) to create a 2x3 matrix composed of random values around 5
>> randp(5,2,3)
ans =
3 7 6
5 2 2
Now type x=randp(5,100,100) to generate a 100x100 matrix of random values with 5 as the central value.
>> x=randp(5,100,100);
Then display the frequency histogram with the hist() function
>> hist(x)
In the histogram, the random values near the value 5 are more frequent than the others.
The gamma distribution
To generate random numbers with the gamma distribution use the command randg()
>> randg(1)
ans = 2.5621
Type randg(100,2,3) to produce a random 2x3 matrix around the value 100
>> randg(100,2,3)
ans =
101.843 98.346 87.956
93.809 99.350 93.122
Now, make a 100x100 matrix with the gamma distribution around the central value 5
Type randg(5,100,100)
>> x = randg(5,100,100)
Finally displays the frequency histogram with the function hist()
>> hist(x)
In the gamma distribution, the values close to the central value are the most frequent.
In this way, you can generate random numbers using different probability distributions.
Note. The rande(), randn(), randp(), and randg() functions have the same parameters as the rand() function. You can use these functions to generate single random values, vectors, or matrices composed of random values.