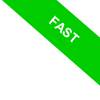
Complex Numbers in Python
In this online lesson, I will explain how to define a complex number in the Python programming language.
What are complex numbers? Complex numbers are an extension of real numbers. Each complex number is composed of a real part and an imaginary part. The real part is a real number, while the imaginary part is the product of a real number and an imaginary constant "i" or "j", called the imaginary unit, which has the property of being a square root of -1. For example, the complex number 2 + 3j is composed of a real part 2 and an imaginary part 3j. $$ z = 2+3j $$ Remember, Python uses only the letter "j" as the imaginary unit. If you use the letter "i", an error will be returned.
Let's take a practical example.
To assign the complex number 2+3j to a variable, you need to type:
>>> num=2+3j
The variable num is assigned the value (2+3j) and the data type <complex>.
Alternatively, to define a variable with a complex number, you can also use the function complex()
num=complex(2,3)
The result is always the same; the variable num is assigned the value (2+3j).
How to check if a variable is complex?
To check the data type, you can type the command type(num).
>>> type(num)
<class 'complex'>
Python confirms that the variable has the data type of the class <class 'complex'>.
Once you have defined two complex variables, you can perform any useful calculation with complex numbers.
Operations with Complex Numbers
For example, assign two complex numbers to two different variables.
>>> a=5+2j
>>> b=4-3j
With these two variables, let's take a look at the main mathematical operations between complex numbers.
Addition
To add the two complex numbers, type a+b
>>> a+b
(9-1j)
The result is the complex number (9-1j).
Note. In operations where you use a complex number, even with a zero imaginary part, the result is always another complex number.
Subtraction
To subtract the two complex numbers, type a-b
>>> a-b
(1+5j)
The result is the complex number (1+5j).
Multiplication
To multiply the two complex numbers, type a*b
>>> a*b
(26-7j)
The result is the complex number (26-7j).
Division
To divide the two complex numbers, type a/b
>>> a/b
(0.56+0.93j)
The result is the complex number (0.56+0.93j).
Conjugate
To find the conjugate of the complex number a=5+2j, use the method conjugate()
>>> a.conjugate()
(5-2j)
The conjugate of 5+2j is 5-2j.
Modulus
To calculate the modulus of the complex number a=5+2j, use the function abs()
>>> abs(a)
5.385164807134504
The modulus of the complex number 5+2j is about 5.38.
Real Part
To extract the real part of the complex number a=5+2j, use the method real
>>> a.real
5.0
The real part of the complex number is 5.0.
Note. When you extract the real or imaginary part of a complex number, the result is always a floating-point real number (float).
Imaginary Part
To extract the imaginary part of a complex number a=5+2j in Python, use the imag method as follows:
>>> a.imag
2.0
This will output the imaginary part of the number, which in this case is 2.0.
Cmath module
Diving into the cmath
module, one uncovers a rich suite of mathematical functions specifically designed for complex number operations.
Magnitude and Phase
When you're looking to extract the magnitude and phase (often referred to as the argument) of a complex number, the cmath module has you covered. By employing the abs() and phase() functions, these values become readily accessible.
import cmath
z1 = 3 + 4j
magnitude = abs(z1)
phase = cmath.phase(z1)
Complex Functions
Beyond the basics, the cmath
module is replete with an array of functions tailored for complex number manipulations. From extracting square roots with sqrt
to computing logarithms via log
, the tools at your disposal are both varied and powerful.
square_root = cmath.sqrt(z1)
logarithm = cmath.log(z1)
Polar and Rectangular Conversions
Transitioning between polar and rectangular forms of complex numbers is a breeze with the cmath
module. Whether you're converting to polar coordinates or reverting to the rectangular form, the module's functions ensure precision and ease.
magnitude, phase = cmath.polar(z1)
z_from_polar = cmath.rect(magnitude, phase)
With this knowledge, you now have a good foundation to begin working with complex numbers in Python.