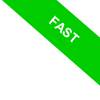
Constants in Java
Let’s dive into the concept of constants in Java. Imagine you need to work with values that remain unchanged throughout the execution of your program.
For instance, consider the number of days in a week, the value of π (pi), or the speed of light. These are values that are constant, and you wouldn’t want to modify them accidentally. In such scenarios, using constants instead of variables is the preferred approach.
In Java, you declare a constant using the `final` keyword.
When you mark a variable as 'final', you're informing the compiler that this variable’s value is immutable once assigned.
Here’s a practical example.
public class ConstantExample {
public static final int DAYS_IN_A_WEEK = 7;
public static final double PI = 3.14159;
public static void main(String[] args) {
System.out.println("A week has " + DAYS_IN_A_WEEK + " days.");
System.out.println("The value of pi is " + PI);
}
}
In this example, there are two constants:
- 'DAYS_IN_A_WEEK' represents the 7 days in a week.
- 'PI' represents the approximate value of π.
By convention, constant names in Java are written in uppercase letters, with words separated by underscores ('_'). This makes it easy to distinguish constants from regular variables.
Once defined, you can use constants throughout your program wherever they’re needed.
Here’s the program output:
A week has 7 days.
The value of pi is 3.14159
In this case, the program simply prints the values of the constants.
Why use constants? First and foremost, constants enhance the readability of your code. For example, if you encounter `7` in the code, its significance might not be immediately clear. However, if you see 'DAYS_IN_A_WEEK', its meaning is instantly understood. By declaring a constant, you ensure that the value won’t be unintentionally altered. Additionally, if the value needs to change, you can do so in one place, rather than searching and replacing every occurrence in your code.
Let’s look at another practical example.
Suppose we need to calculate the circumference of a circle. The formula is:
\[ C = 2 \times \pi \times r \]
In Java, you might implement it like this:
public class Circumference {
public static final double PI = 3.14159;
public static double calculateCircumference(double radius) {
return 2 * PI * radius;
}
public static void main(String[] args) {
double radius = 5.0;
double circumference = calculateCircumference(radius);
System.out.println("The circumference of the circle is: " + circumference);
}
}
In this example, 'PI' is a constant used to calculate the circumference.
Since 'PI' is declared as 'final', you can be confident that the value of π will never be unintentionally altered during the program's execution.
In this case, the program calculates the circumference of a circle with a radius of 5.
The circumference of the circle is: 31.4159
In conclusion, by using constants correctly in your programs, you can prevent errors and make your code clearer and easier to understand for anyone who reads it, including yourself when you revisit it after some time!