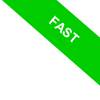
Variables in Java
In this lesson, I'll walk you through how to create and work with variables in the Java programming language.
What are variables? Variables in Java act like containers where you can store different types of data. Before using a variable, you need to specify its type, similar to choosing the right container for a specific object.
To work with variables, you must first declare what type of data they will hold (integers, decimals, characters, strings, etc.).
For example, if you want to store an integer, you would use the `int` type and then assign a name to the variable, such as `int number;`. This declaration means that `number` can only hold integer values.
public class HelloWorld {
public static void main(String[] args) {
// Declaring an 'int' variable called 'number'
int number;
}
}
As we’ll see, besides 'int', Java offers several other primitive data types you can use.
Primitive Types
In Java, there are several "primitive" data types, which are the most basic and fundamental. These are used to store simple data like numbers, characters, and boolean values (true or false).
Here are the primitive types in Java:
- `boolean`: Can hold only two values, `true` or `false`. It's used for making decisions or checking conditions.
- `char`: Stores a single character, like a letter, and uses 16 bits (or 2 bytes) of memory.
- `byte`: Used for very small integers, utilizing 8 bits.
- `short`: For slightly larger integers, requiring 16 bits of memory.
- `int`: The most common data type for integers, using 32 bits of memory.
- `long`: For very large integers, occupying 64 bits.
- `float`: Used for single-precision decimal numbers, with 32-bit memory usage.
- `double`: For double-precision decimal numbers, using 64 bits.
For each primitive type, Java provides an "advanced" version known as a wrapper class. These classes allow you to treat primitive types as objects, which can be beneficial in certain situations.
For example, you can use `Integer` instead of `int` when you need additional functionality that simple primitive types don't provide. Sometimes, Java will automatically convert primitive types to their wrapper classes when needed.
Practical Examples
To declare a numeric variable meant to hold integers (without decimals), you start by writing the int keyword at the beginning of the program.
Then, leave a space and write the name of the variable.
public class HelloWorld {
public static void main(String[] args) {
// Declaring an 'int' variable called 'number'
int number;
}
}
Once you've declared the variable, you can assign it a value.
For example, assign the value 5 to the variable "number".
public class HelloWorld {
public static void main(String[] args) {
// Declaring an 'int' variable called 'number'
int number;
// Assigning a value to the 'number' variable
number = 5;
}
}
Now the variable "number" contains the value 5.
If you reference the variable, you can reuse its content for further operations.
For example, print the variable to the screen using the System.out.println() function.
public class HelloWorld {
public static void main(String[] args) {
// Declaring an 'int' variable called 'number'
int number;
// Assigning a value to the 'number' variable
number = 5;
// Using the 'number' variable to perform an operation
int result = number * 2;
// Printing the result
System.out.println("The result is: " + result);
}
}
This code multiplies the content of the "number" variable by two and assigns the product to the "result" variable.
Finally, it prints the content of the "result" variable. The program's output is:
The result is 10
The content of the variable remains the same as long as the program is running.
When the program ends, the content of the variable is cleared.
Generally, variables are undefined when declared and only acquire a value during assignment or initialization.
int number
If you want to declare multiple variables of the same type, you can list them sequentially, separated by commas.
int number, year
In Java, the assignment operator is the equals symbol '='.
number = 5
The variable name goes on the left side, and the value to be assigned goes on the right.
You can also combine declaration and assignment into a single statement.
int number = 5
If there are multiple variables of the same type, you can also write them like this:
int number = 5, year = 2024;
How to Change the Value of a Variable
You can change the content of a variable at any time. Simply assign it a new value.
For example, after printing it, assign the value 6 to the "number" variable.
Then, print it again.
public class HelloWorld {
public static void main(String[] args) {
// Declaring an 'int' variable called 'number'
int number;
// Assigning a value to the 'number' variable
number = 5;
// Printing the content of the 'number' variable
System.out.println(number);
// Modifying the value of the 'number' variable
number = 6;
// Printing the content of the 'number' variable
System.out.println(number);
}
}
The program's output is:
5
6
How to Create a Floating-Point Variable
To create a floating-point variable, declare it using the double keyword.
For example, declare a variable named "number" as a double.
Then, assign the value 5.5 to the variable.
public class HelloWorld {
public static void main(String[] args) {
double number;
number = 5.5;
System.out.println(number);
}
}
The rest of the program remains the same.
The program's output is:
5.5
The double type allows you to store a value with double precision.
It's ideal for numbers with many digits.
Sometimes it's better to use the float type
If the variable needs to store decimal numbers with fewer digits (such as 5.5), I recommend using the float type, as it requires less memory.
For example, declare the variable "number" as a float. Then assign the numeric value 5.5 to the variable.
public class HelloWorld {
public static void main(String[] args) {
float number;
number = 5.5f;
System.out.println(number);
}
}
In this case, remember to add the 'f' suffix to the end of the number when assigning it.
The program's output is:
5.5
How to Create an Alphanumeric Variable
You can also use variables to store alphanumeric values. To create an alphanumeric variable, declare it using the String keyword.
For example, create a variable of type String and name it "greeting".
Then, assign the string "Hello" to the "greeting" variable.
public class HelloWorld {
public static void main(String[] args) {
String greeting;
greeting = "Hello";
System.out.println(greeting);
}
}
Remember to always enclose alphanumeric values in quotation marks (double quotes).
The program's output is:
Hello
The String type allows you to store sequences of characters (e.g., a name, a code, etc.).
How to Create a Character Variable
If you need to store a single character in a variable, it's better to declare it as a char type, as it uses less memory.
For example, declare the variable using the char keyword and name it "letter".
Then, assign the character 'A' to the "letter" variable.
public class HelloWorld {
public static void main(String[] args) {
char letter;
letter = "Y";
System.out.println(letter);
}
}
In char type variables, remember to always enclose the character in single quotes.
The program's output is:
Y
The char type is designed for single characters and uses less memory compared to a String variable.
Local Variables
When variables are defined within a method, they are known as "local variables" and are only accessible within that context.
They cannot be used outside of that method, making them useful for specific tasks within a portion of your program.
Here’s a simple example that demonstrates the use of a local variable in Java:
public class LocalVariableExample {
public static void main(String[] args) {
// Beginning of the main method
// Declaring a local variable called 'sum'
int sum = calculateSum(5, 7);
// Printing the result
System.out.println("The sum is: " + sum);
}
// Method that calculates the sum of two numbers
public static int calculateSum(int a, int b) {
// Declaring a local variable called 'result'
int result = a + b;
// Returning the result of the sum
return result;
}
}
In the `calculateSum` method, we declare a local variable called `result` with `int result = a + b;`
The local variable `result` is used to store the sum of `a` and `b`, which are the parameters passed to the method. It only exists within `calculateSum` and is not accessible outside of this method.
Once calculated, the value of `result` is returned to the calling method `main` with `return result;`, stored in the `sum` variable, and then printed to the screen.
The sum is: 12
Now you’ve learned how to use variables in Java
In this lesson, I’ve explained how to create and work with numeric and alphanumeric variables in Java.
If you found this lesson helpful, feel free to share it with your friends.