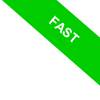
Fields in Java Classes
In Java, every class serves as a blueprint that can hold variables and methods. Fields (or instance variables) represent the data that defines the state of each object created from the class.
What is a field? A field is simply a variable associated with a class or an instance of a class. When you create an object from that class, the object has its own unique values for those fields. Some also refer to them as "properties" or "attributes."
Fields are a key part of Java because they define the state of objects, which in turn influences their "behavior" in the system.
Each object from the same class can have different values for these fields.
Let's look at a simple example: suppose you want to represent a point on a plane using `x` and `y` coordinates.
class Point {
public double x;
public double y;
}
In this case, `x` and `y` are fields of the `Point` class. Every object created from this class will have its own specific coordinates.
Now, let’s create an object from the `Point` class:
Point p1 = new Point();
This object, `p1`, will have its own values for `x` and `y`, independent of any other object created from the `Point` class.
For example, we can assign `p1` the coordinates x=1 and y=2:
p1.x=1;
p1.y=2;
Next, let’s create another object, `p2`, from the same class and assign it the coordinates x=3 and y=4:
Point p2 = new Point();
p2.x=3;
p2.y=4;
These two objects, `p1` and `p2`, are both created from the `Point` class. They share the same fields, `x` and `y`, but hold different values.
System.out.println("p1.x = " + p1.x);
System.out.println("p1.y = " + p1.y);
System.out.println("p2.x = " + p2.x);
System.out.println("p2.y = " + p2.y);
p1.x = 1.0
p1.y = 2.0
p2.x = 3.0
p2.y = 4.0
In the next sections of this guide, we'll dive deeper into field visibility and the different types of fields in Java.
Types of Fields
There are two main types of fields in Java:
- Instance fields
Instance fields belong to a specific instance (object) of the class. This means each object has its own copy of these fields, and their values can differ from one object to another.class Person {
For example, each `Person` object will have its own name and age.
String name;
int age;
} - Static fields
Static fields belong to the class itself, rather than to any particular object. There’s only one copy of these fields, shared among all objects of the class.class Person {
For example, the `numberOfPeople` field is static and keeps track of how many `Person` instances have been created. Every time a new `Person` object is created, this field is incremented.
static int numberOfPeople = 0;
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
numberOfPeople++;
}
}
Field Visibility
Field visibility controls who can access and modify a field. Here are the different visibility levels:
- Public
A field declared as `public` can be accessed from anywhere in the program. This means any other class or method can read or modify its value.class Book {
In this example, the `title` field is public and can be accessed from any part of the program.
public String title;
} - Private
A field declared as `private` can only be accessed within the class where it's defined. This is the most restrictive level of access and is commonly used to protect data by preventing direct access from outside the class.class Book {
In this case, the `title` field is private and can only be accessed or modified via the public `getTitle()` and `setTitle()` methods.
private String title;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
} - Protected
A `protected` field can be accessed by subclasses or classes in the same package. - Package-private (no modifier)
If no visibility modifier is specified, the field is only accessible by other classes within the same package.
Default Field Values
If a field is not explicitly initialized, Java assigns a default value based on its data type:
- Numeric types (`int`, `double`, etc.): 0 or 0.0
- Boolean: `false`
- Objects (e.g., `String`): `null`
For instance, consider this class:
class Book {
String title; // Default value: null
int pages; // Default value: 0
}
Since you haven’t explicitly assigned values to the fields, Java will assign `null` to the `title` field and `0` to the `pages` field.
Final Fields
A field declared as `final` cannot be changed once it has been assigned a value.
In other words, its value can only be set once, typically during its declaration or in the constructor.
Here’s an example of a class that uses a `final` field:
class Book {
final String ISBN;
Book(String isbn) {
this.ISBN = isbn; // The ISBN can only be assigned once
}
}
In this case, the ISBN can only be set once, and it cannot be changed afterward.
Encapsulation
Finally, let's discuss encapsulation, a core concept in object-oriented programming.
The idea behind encapsulation is to hide an object’s internal details, allowing access only through public methods that manage interactions with the internal state.
To achieve this, fields are declared `private`, and public `getter` and `setter` methods are used to access or modify them.
class Person {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
In this example, the `name` field can’t be modified directly from outside the class. Instead, it can only be updated through the `setName()` method, giving you more control over when and how the field is changed.