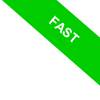
Java Classes
In this lesson, I’ll walk you through how to write a class in Java. To create a class, you use the class keyword.
class className() {
// variables and methods
}
The class name must be unique within the program. Inside the curly braces, you define the class’s variables (attributes) and methods.
- Attributes describe an object’s properties, like its color or size.
- Methods represent actions the object can perform, like calculating a square root.
A class serves as the blueprint for an object. When you create a class, you’re telling the computer, "This is the model of something I’m going to use, and this model has specific characteristics and abilities."
Once the class is defined, you can use it to create objects, which are concrete instances of that class.
Still unsure what a class is? Think of it like a cookie cutter. The cutter gives you the shape, but until you fill it with dough, you don’t have an actual cookie. Similarly, a class defines what an object should do or how it’s structured, but it’s not a physical object yet. The class is the cutter, and the cookie is the object.
A Practical Example
Let’s define a class called `Automobile`.
class Automobile {
// Attributes (characteristics)
String make;
String model;
int year;
// Constructor (how to create an Automobile object)
public Automobile(String make, String model, int year) {
this.make = make;
this.model = model;
this.year = year;
}
// Method (what the Automobile can do)
public void startEngine() {
System.out.println("The engine is on!");
}
}
The class defines three attributes: `make`, `model`, and `year`, which describe the car’s characteristics.
It also includes a method called `startEngine()`, representing an action the car can take, such as starting the engine.
One of the great things about classes is that they don’t just describe what an object is—they also define what it can do! Methods like `startEngine()` bring the object to life. It’s like pressing the “start” button on a car.
After defining the `Automobile` class, you can use it to create real cars (objects) based on this model.
public class Main {
public static void main(String[] args) {
// Create an Automobile object
Automobile myCar = new Automobile("Toyota", "Corolla", 2020);
// Use the object's methods
myCar.startEngine(); // Prints: The engine is on!
}
}
In this example, you’ve created an object called `myCar`, which represents a specific vehicle: a 2020 Toyota Corolla.
Notice how we used the constructor, which is a special function named after the class, to "build" the car.
Then, we called the `startEngine()` method on the object, which prints the message:
Prints: The engine is on!
You might be wondering, "What’s that `this` keyword?" It’s straightforward. Imagine you’re explaining who you are, and you want to make it clear that your name refers to yourself, not someone else. In Java, `this` works similarly—it refers to the current object. So, when I write:
this.make = make;
I’m saying, "Assign the value you provided to this object's `make` attribute."
Example 2
Here’s another example: let’s define a class `Calculator` that can perform mathematical operations.
class Calculator {
// Method to add two numbers
public int add(int a, int b) {
return a + b;
}
// Method to subtract two numbers
public int subtract(int a, int b) {
return a - b;
}
}
Now, you can create an instance of this class and start using your virtual calculator to perform calculations!
public class Main {
public static void main(String[] args) {
// Create a Calculator object
Calculator calc = new Calculator();
// Use its methods
int sumResult = calc.add(10, 5); // Returns 15
int subtractResult = calc.subtract(10, 5); // Returns 5
System.out.println("Sum result: " + sumResult);
System.out.println("Subtraction result: " + subtractResult);
}
}
Sum result: 15
Subtraction result: 5
Now, with a bit of imagination, you can create anything you want, step by step! Whether it’s cars, calculators, or even virtual spaceships, it all starts with classes.
The key is to enjoy figuring out how things work. Once you grasp the fundamentals, like classes in Java, everything else is just about experimenting and building!