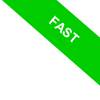
Debugging a Matlab script
Let's talk about debugging a script in Matlab.
What is debugging? Debugging is the process of searching for an error in your script. The term "bug" refers to a problem or error in the code. It's called that because in the early days of computing, an actual insect (a bug) inside a computer could cause malfunctions. Nowadays, that's not really an issue, but the term "bug" is still used to indicate a program malfunction.
So, how do we debug a script in Matlab? Well, we can use the "keyboard" statement.
keyboard
The term "keyboard" is a breakpoint that allows you to suspend the execution of a script to check or modify the value of environment variables.
It's really useful for debugging and testing. From there, you can perform various operations.
How does the "keyboard" command work?
When Matlab encounters the "keyboard" keyword, it suspends the script execution and opens a debug prompt.
The debug prompt looks like this:
k>>
At this point, you can perform several operations. For example, you can check or modify the value of variables in the operating environment.
Once you've made the necessary changes, you can continue the execution of your script using the dbcont command.
Alternatively, you can use the dbstep command to execute only the next instruction of your script in step-by-step mode.
Note. If you want to exit the debug mode and terminate the execution of your script, you can use the "dbquit" command. In that case, the script won't resume execution at the point where it was interrupted.
Let me give you a practical example.
Suppose we have this script:
x=2;
y=3;
disp(x+y)
When you execute this script, the output is:
5
Now, let's modify the script by inserting the "keyboard" keyword before the display command.
x=2;
y=3;
keyboard
disp(x+y)
When you execute the script now, the execution will pause when the interpreter encounters the "keyboard" keyword, and the debug prompt will appear.
k>>
Note. If you're using the Matlab graphical interface, you'll see the debug commands at the top of the window, which allow you to continue execution or execute the next instruction step-by-step.
From the debug prompt, you can modify the value assigned to the variable "x".
Just type x=4 and press Enter.
k>> x=4
Then, execute the dbcont command to continue the execution of the script.
k>> dbcont
The script will resume execution at the point where it was interrupted, but the value of "x" will have changed to 4.
The script will add x=4 and y=3, returning the result x+y=7.
7
This allows you to modify the variables and parameters of a program during execution.
You can use this technique to search for errors, experiment with solutions to a problem, or improve the efficiency of your script without having to modify the code every time.