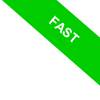
Generating Random Numbers in MATLAB
In this tutorial, I will explain how to generate random numbers in MATLAB.
What are random numbers? They are numbers that are extracted randomly from a numerical set. In computer science, they are also known as random numbers. More specifically, they are pseudo-random numbers because there is a specific algorithm used to generate them.
To generate a random number in MATLAB, you can use the rand() function.
The rand() function generates a real number between 0 and 1.
>> rand()
ans = 0.8147
If you want to generate a real number between 0 and 10, you can type rand()*10
>> rand()*10
ans = 9.0579
If you want to generate a real number between 0 and 100, you can type rand()*100
>> rand()*100
ans = 12.6987
To generate a random real number between 18 and 30, you can type rand()*12+18
>> rand()*12+18
ans = 28.9605
How to generate random integers?
To generate a random integer, you can round the result of the rand() function using the round() function.
>> round(rand()*10)
ans = 6
Alternatively, you can use the randi() function to generate random integers.
This function generates an integer between 0 and the maximum value specified in parentheses.
For example, the randi(10) function generates an integer between 0 and 10.
>> randi(10)
ans = 6
To generate a random integer between 18 and 30, you can type randi(12)+18
>> randi(12)+18
ans = 23
Alternatively, you can specify the numerical range in square brackets as randi([18,30])
>> randi([18,30])
ans = 25
How to generate a vector of random numbers?
To create a vector of random real numbers, you can use the rand() function and specify the number of rows and columns of the vector in parentheses
For example, to generate a random vector with three elements arranged in a column, you can type rand(3,1)
>> rand(3,1)
ans =
0.9157
0.7922
0.9595
To generate the same vector with three elements arranged in a row, you can type rand(1,3)
>> rand(1,3)
ans =
0.6557 0.0357 0.8491
To create a vector of random integers between 1 and 10, you can type randi(10,3,1)
The first parameter (10) is the maximum value, while the second (3) and third (1) parameters are the number of rows and columns of the array.
>> randi(10,3,1)
ans =
8
4
7
How to generate a matrix of random numbers?
Similar to vectors, you can also generate a matrix of random numbers.
For example, you can type randi(10,3,3) to obtain a square 3x3 matrix with random integer numbers arranged in three rows and three columns.
>> randi(10,3,3)
ans =
2 3 9
8 1 7
1 1 4
To generate the same matrix with real numbers, you can type rand(3,3)*10
>> rand(3,3)*10
ans =
9.5022 3.8156 1.8687
0.3445 7.6552 4.8976
4.3874 7.9520 4.4559