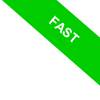
Reading and writing a text file in Octave
In this tutorial, I will explain how to write and read a text file using Octave.
How to write a new file
To write a new file, use the fopen() function, indicating the file name and the write access mode "w". For example:
>> MyFile=fopen("test2.txt", "w");
Then, write two records in the file using the fprintf() function:
>> fprintf(MyFile, "1 record \n");
>> fprintf(MyFile, "2 record \n");
Alternatively, you can also write the records with the fputs() function:
>> fputs(MyFile, "1 record \n");
>> fputs(MyFile, "2 record \n");
The result is the same.
Note. At the end of each string, add the newline symbol \n to go to a new line and start a new record. If you don't add it, the next printf() statement will continue writing in the same record.
When you finish writing the records, close the file using the fclose() function:
>> fclose(MyFile);
How to read a file
To read a text file, use the fopen() function, indicating the file name and the read attribute "r".
Assign the file to a transit variable, such as MyFile
>> MyFile=fopen("test2.txt", "r");
Remember that the file has two records within.
To read a record from the file, use the fgetl() statement:
>> fgetl(MyFile)
This statement reads the content of the first record
ans = 1 record
To read the second record, which is also the last one., use the fgetl() statement again:
>> fgetl(MyFile)
The command retrieves the content of the file's second and final record
ans = 2 record
If you type the fgetl() command again, it will return -1 because the file has reached the end.
>> fgetl(MyFile)
ans = -1
When you're done, close the file.
>> fclose(MyFile);
How to read the file using a loop
You can also read the contents of the file using a loop structure, without having to type fgetl() each time
MyFile=fopen("test4.txt", "r");
eof=0;
while eof==0
rec =fgetl(MyFile)
if (rec==-1) eof=1; endif
end
fclose(MyFile)
How to add records to an existing file
To add more records to an existing file, open the file in append mode using the fopen() function with the access mode 'a'.
For example, to open the "test2.txt" file, which already has two records.
>> MyFile=fopen("test2.txt", "a");
Then, add a new record with the fprintf() or fputs() function.
>> fprintf(MyFile, "3 record \n");
When you finish adding more records, close the file for writing with the fclose() function
>> fclose(MyFile);
Now there are three records in the file.
File access modes
In addition to the read ('r') and write ('w') access modes, there are other modes:
'r' = open the file in read mode
'w' = open a new file in write mode
'a' = add records to the end of an existing file
'r+' = open a file in read and write mode
'w+' = open a new file in read and write mode
'a+' = open a file in read and write mode at the end of the file
In this lesson, you have learned how to read and write a text file using Octave.