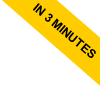
Command Line Argument Parsing in Python
In this Python course segment, let's delve into the art of parsing command line arguments.
Understanding Argument Parsing: Running a Python script often involves passing specific parameters. These are interpreted and processed through what's known as parsing. Essentially, parsing breaks down and analyzes the string of parameters (or arguments) for the script to utilize.
Consider a basic script, named greet.py, designed to output "Hello" followed by a person's name.
The twist here is that the user specifies the name as a parameter when running the script.
greet.py Tom
Our goal is to enable the "greet.py" script to intelligently read the "Tom" parameter passed externally. This is achieved through parsing, using the sys module.
Embed this code into a script and save it as "greet.py" on your computer.
- import sys
- # Verify if a name argument is provided
- if len(sys.argv) > 1:
- # Extract the first command line argument (excluding the script name)
- name = sys.argv[1]
- print(f"Hello, {name}!") # Outputs the personalized greeting
- else:
- print("Please provide a name as an argument.")
Python automatically stores parameters (or arguments) in a system list named sys.argv.
Keep in mind, these parameters are stored as strings. So, if your input is numeric, it will need to be converted within the script.
After saving your script, execute it from the command line, inserting any name as an argument.
python greet.py Tom
Make sure to separate the script name "greet.py" and the argument "Tom" with a space. The script then executes the parser, prompting it to display "Hello, Tom!" on your screen.
Hello Tom!
Try running the script with a different name, such as "Mary".
python greet.py Mary
This time, the script will show "Hello, Mary!"
Hello Mary!
If you execute the script without any arguments, it prompts you to input a name.
This example, though straightforward, effectively demonstrates the concept and functionality of argument parsing in Python.
Passing Multiple Arguments
Handling multiple arguments is a breeze. You simply retrieve them using their respective indices.
Let's modify the greet.py script to accept a name and an integer:
- import sys
- # Ensure two arguments are provided: a name and a number
- if len(sys.argv) == 3:
- # Retrieve the first argument, which is the name
- name = sys.argv[1]
- try:
- # Attempt to convert the second argument into an integer < li>number = int(sys.argv[2])
- # Display the message incorporating the provided information
- print(f"Hello, {name}! You entered the number {number}.")
- except ValueError:
- print("Please provide a valid number.")
- else:
- print("Please input exactly two arguments: a name and a number.")
This refined script ensures that exactly two arguments are provided, as indicated by len(sys.argv) == 3.
If this condition is met, the script attempts to convert the second argument into an integer. Should the conversion fail, a clear error message is displayed.
If the script does not receive the correct number of arguments, it responds with an appropriate error message.
Note that len(sys.argv) == 3 is used instead of 2 because Python's sys.argv list includes the program name as its first element. So, even with no arguments passed, sys.argv
always contains at least one element. The first user-provided parameter is at index 1, the second at index 2, and so forth.
Now, test this script by executing it with two command line arguments:
python greet.py Tom 5
The script will then eloquently display:
Hello, Tom! You entered the number 5.
This approach allows you to craft scripts capable of handling multiple arguments. As we progress through our course, we'll explore even more sophisticated methods for parsing command line arguments.