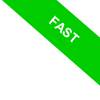
Python Strings
Strings in Python are one of the fundamental data types of the programming language and are used to represent a sequence of characters.
Strings are objects that belong to the category of sequences, like tuples and lists, because they are ordered containers. Each character in the string is associated with an index number that goes from left to right. Therefore, the first character has index 0, the second character has index 1, and so on.
To create a string in Python, you can enclose the text between either single or double quotes. For instance:
>>> var = 'This is a string'
Alternatively, you can also use double quotes to create a string.
>>> var = "This is a string"
The final result is the same.
Note: Always remember to use the same type of quotes at the beginning and end of the string.
If the string is very long and spans across two or more lines of code, you can use three quotes to enclose it. For example:
>>> var = """This is a string
that spans across two lines"""
Once created, you can check the type of the object using the type() function.
>>> type(var)
Python will respond that the object belongs to the "str" class of strings.
<class 'str'>
In Python, the string object has several useful methods that allow you to manipulate the string in various ways, such as concatenation, splitting, and searching for substrings.
Indexing
Indexing allows you to access a single character of the string by specifying its index (position) within square brackets. For instance:
>>> string = "This is a string"
>>> string[0]
Python reads the character at index 0, which is the first character of the string.
In this case, it is the character "T" that has index 0.
T
You can use indexing to read any character of the string.
For example, type string[10]
>>> string[10]
Python returns the character at position index 10 of the string, which is "s".
s
Note. Indexing is common to all objects that belong to the category of sequences, because in turn, this category is part of the category of iterable objects. All iterable objects have the characteristic of being unpacked into single elements associated with labels.
The index() Method
The index() method finds the position of the first occurrence of the element that you pass as an argument.
For example, type this code:
>>> string = "This is a string"
>>> string.index('s')
In this case, the string.index('s') method searches for the first occurrence of the character 's' inside the string.
It finds it at position index 3
3
In fact, the character 's' is the fourth character of the string, and it is located at index position 3.
Note. Remember that in Python, indices start from 0. Therefore, the first character of the string has index 0, the second character has index 1, the third character has index 2, the fourth character has index 3, the fifth character has index 4, etc.
Concatenation
To concatenate two strings, you can use the + operator.
For example, type this code:
>>> string1 = "This is"
>>> string2 = "an example"
>>> string = string1 + " " + string2
The result is a string that contains the sequence of characters "This is an example."
The split() Method
The split() method allows you to break the string into multiple parts (substrings).
For example, type this code:
>>> string = "This is a list of words"
>>> words = string.split(" ")
This code splits the string using the whitespace character " " as a separator and generates an array that contains the words in the text:
["This", "is", "a", "list", "of", "words"]
The find() method
The find() method allows you to search for a substring within a string.
This method returns the index of the first occurrence of the substring within the main string.
For example, consider the following code:
>>> string = "This is a string"
>>> string.find("is")
In this case, the method outputs the number 2 because the substring "is" begins at the 2th position of the main string.
It finds it at position index 2
2
Slicing
Slicing allows you to extract a substring from a string.
For example, consider the following code:
>>> string = "This is a string"
>>> string[5:7]
When you enter string[5:7], the Python interpreter extracts the substring that begins at index position 5 of the string up to position 7 (excluding it).
This example of indexing results in the substring "is".
is
These are just a few practical examples of using strings in the Python language, but there are many others.
Strings are highly versatile and can be used for a wide range of applications.
Understanding Text and Byte Strings in Python
Python offers two distinct string types:
- Text Strings (str)
Text strings, identified as str type, are utilized for representing alphanumeric textual information. They can be easily defined using a set of quotes, either single or double.
test="example"
print(type(test))<class 'str'>
- Byte Strings (byte)
Byte strings, categorized as byte type, extend beyond handling textual data. They are distinguishable from text strings by the presence of a 'b', either in lowercase or uppercase, prefacing the string.
test=b"example"
print(type(test))<class 'byte'>
Distinguishing Between Text and Byte Strings
While text strings are composed of straightforward, visible characters, byte strings represent characters through byte sequences, with each byte expressed in hexadecimal format.
This distinction allows text strings to be versatile, capable of storing not only conventional text but also special characters and characters from various alphabets.