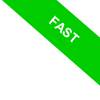
How to make a loop in Python
In this online lesson I'll explain how to make a loop in the Python language.
What is a loop? It is an iterative structure that repeats the execution of a code several times until an exit condition occurs. It's also called "cycle".
In the python language you can create a loop using the for and while statements.
For statement
The for function loops that iterates for a predefined number of times.
Example
Here is a practical example of a loop made with the for statement
This script runs ten iterations and prints the numbers 1 to 10 on the screen.
1
2
3
4
5
6
7
8
9
10
When you use the iterative structure for you indicate precisely how many iterations it has to do
If you don't know how many iterations the loop will have to do, use the while statement.
While statement
The while function allows you to create a loop that iterates until a condition is true.
Example
For example, this script generates a random number from 1 to 10 in each iteration.
If the random number is not five, it performs another iteration.
A possible output of the program is
3
1
6
8
2
5
In this case you cannot know the number of iterations in the loop. In the best case it is only one but in the worst case they are infinite.
To avoid infinite loops I recommend adding a forced exit condition from the loop by a break statement.
Example
For example, this script ends the loop after 100 iterations if random number five has not been generated
Therefore, in the worst case, the script runs one hundred iterations.
If you like this Nigiara lesson, share it with your friends.