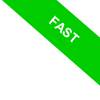
How to generate a random number in Python
In this lesson I'll explain how to program a random number generator in the python language.
Python doesn't have a random function.
For the generation of random numbers you have to import the random module.
import random
In the random module there are several functions to generate one or more random numbers.
The most used and common functions are the following:
- random.random()
generates a real random number from 0 to 1 - random.randint(x,y)
generates a random integer from x to y
I'll give you a practical example
This script generates a random number from 0 to 1
import random
x=random.random()
print(x)
The random() function generates a random real number (e.g. 0.88789241500074575) and writes it to the variable x
0.8878924150007457
This other script generates an integer random number from 1 to 10
import random
x=random.randint(1,10)
print(x)
The randint() function generates a random integer number (e.g. 5) and writes it to the variable x
5
If this python online lesson is useful, please keep following us.