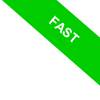
Scilab Variables
In the realm of programming, variables serve as the backbone, acting as symbolic placeholders for data. Let's delve into how Scilab, a powerful computational tool, handles these essential elements.
In Scilab, you can define a variable simply by assigning a value to a name. There's no need to declare the data type beforehand.
The assignment operator is the equal sign (=).
variableName = value
At its core, a variable in Scilab is a symbolic name you assign to a specific piece of data, be it numbers, strings, or matrices. The beauty of Scilab lies in its simplicity: you can create a variable just by pairing a name with a value. No need to fuss over data types upfront; Scilab's got you covered.
Here are some hands-on examples of using variables.
Define three variables with the names "a", "b", and "c".
a = 5;
b = 3.14;
c = 'Scilab';
Notice the diversity? 'a' is an integer, 'b' is a floating-point number, and 'c' is a string.
Scilab's intuitive design automatically discerns the data type based on the value you assign.
For those who love efficiency, Scilab allows multiple assignments in one go by separating the assignments with commas.
a=5 , b= 3.14 , c = 'Scilab'
A pro-tip: always cap off your assignments with a semicolon (`;`).
It keeps your console neat by preventing Scilab from echoing the assignments.
a = 5;
If you omit the semicolon, Scilab will display the assignment in the console.
For instance, type a=5 without the semicolon and hit enter:
a=5
The outcome remains the same, but in this case, Scilab displays the variable name, the equal sign, and the assigned value in the console:
a =
5.
This redundant information can slow down execution, unnecessarily consume computational resources, and clutter the console, making it harder to read essential details.
What's the purpose of variables?
Think of variables as the workhorses of your code. Once defined, they can be woven into mathematical expressions, replacing direct numbers for more dynamic computations.
For example, assign integer values to variables "a" and "b", then add the values and store the result in variable "c".
a=5
b=2
c = a + b;
Variable Naming Conventions
When christening your variables, adhere to these guidelines:
- The name of a variable should not begin with a number.
- The rest of the name can consist of letters, numbers, and the underscore "_" character.
- Scilab is case-sensitive, meaning it distinguishes between uppercase and lowercase letters. So, "data" and "Data" are two distinct entities.
Steer clear of Scilab's reserved keywords to avoid any hiccups.
Changing a variable's value and data type
Already assigned a value to a variable? Feel free to change it up, even switching data types if needed.
For instance, you can assign an integer to a variable and then immediately assign a string to it.
a=5;
a='Scilab'
Scilab only considers the most recent value assigned to the variable.
To declutter, use the clear command. It wipes a variable's content, freeing up your workspace.
For example, assign a string to the variable "name"
name = 'Scilab'
Then, clear the variable's content using the 'clear' command followed by the variable's name:
clear name
This action removes the "name" variable from the workspace.
If you wish to clear all the variables in the workspace at once, simply type the 'clear' command without specifying a variable name:
clear
Data Types in Scilab
Scilab supports various data types, including:
- Integers
Both the positives and the negatives.
- Floating-point Numbers
These include decimal numbers. - Strings
Think character sequences. - Arrays
Collections of values, spanning vectors to tensors. - Lists
Ordered item collections.
Additionally, Scilab offers predefined variables like %pi (π's value) and %e (base of natural logarithm). These are invaluable for mathematical computations.
These are handy for utilizing mathematical constants without having to compute them.
Difference between global and local variables
In Scilab, variables can either be global or local.
- Global Variables
These are accessible from any part of the program. You can define a global variable using the 'global' statement. - Local Variables
These are confined to a specific function or code block.
In conclusion, Variables are the linchpin of Scilab programming, offering a dynamic way to store and manipulate data. Mastering them is pivotal for anyone keen on harnessing Scilab's full potential.