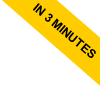
Anonymous Functions in Matlab
Let's talk about anonymous functions in Matlab.
What is an anonymous function? An anonymous function is a mathematical function with one or more variables that you define without giving it a name inside other functions. They are called "anonymous" because, well, they don't have a name. You can only use them inside the composed functions they are in.
To create an anonymous function in Matlab, you use this syntax:
name@(variables) expression
First, you write the symbol "@" followed by the independent variables of the function between parentheses.
Then, after a space, you write the expression of the function.
Now, why would you want to use an anonymous function, you might ask? They come in quite handy when you need to create a routine that you will use only once within a script. This way, you avoid having to define another function in the script, making the script more compact and occupying less memory.
Let me give you a practical example.
Suppose you want to square the elements of an array. You could do this with the arrayfun() function, which uses an anonymous function to square each element of the array. The function looks like this: @(x) x^2.
A = [1, 2, 3, 4, 5];
B = arrayfun(@(x) x^2, A);
disp(B);
Of course, the anonymous function doesn't have a name.
You can only use it inside the arrayfun() function. Outside of it, it is not visible.
The result is saved in the variable B and printed using the disp() function.
1 4 9 16 25
These are the squares of the elements of the vector A.
Now, you can also use anonymous functions as regular functions, but in this case, you have to give them a name before the "@" symbol.
For instance, consider the following anonymous function:
>> f = @(x,y) x^2+y^2
This function is called "f" and has two independent variables, x and y, with the expression x2+y2
$$ f(x,y) = x^2+y^2 $$
Note. In this example, the function is called "f," but it can be called anything else.
After defining the function, you can invoke it multiple times with different parameters.
For example, you can type f(2,3) and get the result of the expression x^2+y^2.
>> f(2,3)
The function receives the parameters x=2 and y=3 and assigns them to the independent variables.
Then, it calculates and displays the result of the expression x2+y2, which is 13.
ans = 13
The result of the function is 13 because by performing the expression with the assigned values, you get 13.
$$ f(x=2,y=3) = x^2 + y^3 = 2^2 + 3^2 = 4+9 = 13$$
Now, invoke the function again by passing it different parameters.
For example, type f(3,4).
>> f(3,4)
The function receives the parameters x=3 and y=4 and assigns them to the variables x and y.
It performs the calculation of the expression and returns 25.
ans=25
The result of the function is 25 because:
$$ f(x=3,y=4) = x^2 + y^3 = 3^2 + 4^2 = 9+16 = 25 $$
Overall, anonymous functions can be quite useful in Matlab when you want to define a function without having to give it a name or when you want to use a function only once within a script. So, give them a try and see for yourself.