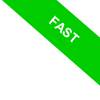
Functions in Matlab
Let's talk about how to create a function in Matlab, shall we?
So, what is a function? It's a piece of code in a program that you can execute whenever you need it. The code of a function is separated from the main program, and it can be located in the same file as the program or in a different file. The use of functions allows you to make the code of a program more concise and orderly.
To define a function in Matlab, you need to follow this syntax:
function [y1, y2, ... ] = function_name([x1,x2,...])
code to be executed
end
- The letters x1,x2... represent the input variables that the function receives from the call.
- The letters y1,y2... represent the output variables that the function returns to the calling program.
In Matlab, functions must always be added to the last lines of a script. Otherwise, the script will give you an error.
Once you have defined a function, you can call it from different parts of the program and execute it several times.
Example
Let me give you a practical example. Suppose you want to write a new script and create a function called "average()".
This function should receive two numerical data x1 and x2 as input and return their arithmetic mean y as output.
Here's how you can define the function in Matlab:
function y = average(x1,x2)
y = (x1+x2)/2;
end
Now, you can insert a call to the "average()" function in the code of the main program. For example:
m=average(2,4);
disp(m);
function y = average(x1,x2)
y = (x1+x2)/2;
end
The function "average()" receives the two input values x1=2 and x2=4, calculates their average y=(2+4)/2=3, and returns the result y=3 to the calling program.
The calling program receives the output value of the function (3) and assigns it to the variable "m" (m=3).
Finally, the program prints the value of "m" on the screen.
3
If you want, you can also make another call to the "average()" function in the main program, passing different values as input. For example:
m=average(2,4);
disp(m);
m=average(4,6);
disp(m);
function y = average(x1,x2)
y = (x1+x2)/2;
end
- Note. As you can see, the program calls the "average()" function twice from two different points in the main program.
- The first time, it passes the values 2 and 4, receiving 3 as the answer.
- The second time, it passes the values 4 and 6, receiving 5 as the answer.
This time, the function "average()" receives the input values x1=4 and x2=6, calculates their average y=(4+6)/2=5, and returns the result y=5 to the calling program.
The calling program receives the output value of the function (5), assigns it to the variable "m" (m=5), and prints the value of "m" on the screen.
Therefore, this time the output is 5.
3
5
So, as you can see, defining and using functions in Matlab is quite simple and straightforward.
It allows you to write more efficient and organized code, which is always a good thing in any programming language.
How to return multiple output values
In Matlab you can actually return more than one value from a function. It's quite nifty, really.
Let me show you an example.
Say we have this function called "power" that takes a single input value, and produces two output values.
[y1,y2]=power(2);
disp(y1);
disp(y2);
function [y1, y2] = power(x)
y1=x^2;
y2=x^3;
end
In this case, the call [y1,y2] = power(2) passes a single input value x=2 to the function.
The power() function calculates the square x2=4 and cube x3=8 of the variable x=2, and returns them to the calling program.
The calling program receives the two output values, assigns them to the variables y1=4 and y2=8, and finally prints them to the screen.
4
8
The return statement
But what if we want to exit the function early, before it finishes executing all the statements in the code block? That's where the "return" statement comes in handy.
The return statement allows to exit a function early.
When Matlab encounters the return statement in the code block of a function, it terminates the execution of the function and returns control to the calling program.
Note. If the return statement is not present in the code block, control automatically returns to the calling program after the execution of the last statement in the function block.
For instance, take a look at this modified version of the "power" function:
[y1,y2]=power(-2);
disp(y1);
disp(y2);
function [y1, y2] = power(x)
if x<0
y1=0
y2=0
return
end
y1=x^2;
y2=x^3;
end
Here, we've added an "if" statement to check if the input value is negative.
If it is, we set the output values to 0, and then immediately exit the function using the "return" statement.
This allows us to return early without executing any more statements in the code block.
In this sample code, the passed value is negative (x<0), the return statement terminates the execution of the power() function, returning the results y1=0 and y2=0.
0
0
So there you have it, the "return" statement can be quite useful for exiting functions early. Just remember to use it wisely, and only when necessary.