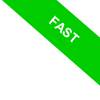
Global Variables in Matlab
Let's talk about global variables in Matlab.
What is a global variable? Now, a global variable is a variable that can be accessed from anywhere in your program, and that includes functions in your script even if it's not explicitly passed as a parameter.
Let me give you an example of how to define a global variable.
To define it, you simply use the 'global' command followed by the variable name. For instance:
>> global a
This command defines a new global variable named "a".
Once you've defined it, you can assign it a value using the assignment operator. For instance:
>> a=1;
Now, let's say you want to create a function that uses the global variable "a".
To do so, you simply need to include the 'global' command for "a" at the beginning of your function. Here's an example:
>> function f()
global a
disp(a)
end
This function will access the global variable "a" even if it's not passed as a parameter. To test it out, just call the function:
>> f
a = 1
And you'll see that it will display the value of "a", which is currently 1.
How to modify the value of a global variable?
Now, what if you want to modify the value of the global variable "a"? Well, that's easy.
Once you've initialized it, you just need to assign it a new value using the assignment operator. Here's an example:
>> global a;
>> a=1;
>> a=2;
This will update the value of the global variable "a" to 2, and any function in your script can access this new value.
How to delete global variables?
Lastly, let's talk about deleting global variables. If you want to remove all global variables from the workspace, just type the command 'clear global'. For instance:
>> clear global
This will delete all global variables while leaving other variables intact.
And if you want to delete a specific global variable, just type 'clear global' followed by the variable name. For instance:
>> clear global myVar
This will delete the global variable named "myVar" from the workspace.