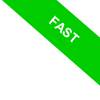
Matlab arrays
In this lesson, I'll walk you through how to define an array in Matlab.
But first, what exactly is an array? Simply put, an array is an ordered collection of two or more data values. Unlike variables, arrays can hold multiple data values within them. These data values can be numeric, alphanumeric, Boolean, or anything else you might need. Arrays are structures that are very similar to vectors and matrices in mathematics.
Let me give you a practical example.
First, write the name of a variable on the left side of the equal sign.
Next, on the right side of the equal sign, write some numbers enclosed in square brackets, separating them by commas. For instance:
>> vector = [1, 2, 3, 4, 5]
This creates an array variable containing a vector of five elements arranged in a row.
vector =
1 2 3 4 5
Using a space instead of a comma to separate the elements will yield the same result.
The final outcome will remain unchanged.
>> vector = [1 2 3 4 5]
vector =
1 2 3 4 5
Now separate the elements of the array using the semicolon symbol as the delimiter.
The resulting variable is an array containing a column vector with five elements arranged vertically.
>> vector = [1; 2; 3; 4; 5]
vector =
1
2
3
4
5
Note. In linear algebra, row vectors and column vectors are not interchangeable. For instance, it is possible to add two row vectors or two column vectors together only if they have the same elements. However, it is not possible to add a row vector to a column vector since they have different arrangements.
In Matlab, arrays can contain not only numerical values but also strings and other alphanumeric data.
To include alphanumeric elements in an array, you need to enclose them in double quotes or double apostrophes. For example:
>> letters = ['A', 'B', 'C', 'D']
On the other hand, when the array elements are strings - that is, sequences of characters - it is more convenient to enclose them in curly braces. For instance:
>> cities = [{"London", "Paris", "Rome", "Madrid"}]
The output of this code is an array called 'cities', which contains four alphanumeric elements.
cities = London Paris Rome Madrid
You can use arrays to define matrices in Matlab as well.
For instance, you can create a matrix with 2 rows and 3 columns by writing M=[1 2 3; 4 5 6].
>> M = [ 1 2 3 ; 4 5 6 ]
M=
1 2 3
4 5 6
The output displays a rectangular matrix consisting of six numerical elements.
$$ M = \begin{pmatrix} 1 & 2 & 3 \\ 4 & 5 & 6 \end{pmatrix} $$