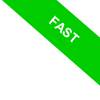
Variables in Matlab
So you want to learn about variables in Matlab? Well, let me tell you, it's like having a box where you can store a piece of information.
What is a variable? Variables are memory addresses where you can record data, whether it's a numerical value or a string of characters. Once you've stored the data in a variable, you can call it and use it in your calculations.
To define a variable in Matlab, you need to give it a name.
After the variable's name, you'll use the equal sign (=) to assign a value to the variable.
variableName = value
For example, let's create a variable named "year" and assign it the value of 2020:
>> year = 2020
And just like that, Matlab has added a variable named "year" to the workspace with the numeric value of 2020.
Now, if you type:
>> year + 1
Matlab will read the value stored in the "year" variable (which is 2020) and increment it by one (2020+1).
The resulting output will be:
ans = 2021
Now, you may be wondering if variables can only store numerical values. The answer is no.
You can also use variables to store strings, boolean values, or other data types. If you want to assign a string to a variable, be sure to enclose it in double quotes or single quotes. For example:
>> name = 'Nigiara'
or
>> name = "Nigiara"
Generally, a variable can store integer or real numbers, characters, logical boolean values (1 or 0), complex numbers, and more.
Here are a few practical tips for using variables in Matlab
When you assign a value to a variable, Matlab will display the value you assigned on the command window.
>> year = 2020
ans = 2020
>>
To avoid this repetition, add a semicolon (;) at the end of the command.
>> year = 2020;
>>
If you want to assign multiple variables on the same line, separate each assignment with a comma.
>> a=3 , b=5 , c=7
You can also use a semicolon as a separator after each assignment to avoid printing the output on the command window.
>> a=3; b=5; c=7;
What names should you use for variables?
You can choose any name for variables, but you must respect some of Matlab's rules:
- A variable name must begin with a letter
The name can only contain letters and/or numbers, and optionally an underscore (_). - You can use lowercase or uppercase letters
The variable name can be lowercase or uppercase, but Matlab is case sensitive, so "year" and "YEAR" are two different variables. - Do not use special characters or symbols in variable names, except for the underscore (_).
The name of a variable must not contain special characters (such as &, $, #, etc.). The only exception to the rule is the underscore _ which you can use to compose the variable name. - Do not use Matlab keywords as variable names
The name of a variable cannot coincide with a keyword already used by Matlab.
Note. To check if a name is a Matlab keyword, you can use the iskeyword() function. Simply insert the name of your variable between two quotes within the parentheses. If the function returns 0, you can use the name for your variable because it is not a keyword.
But if it returns a 1, then the name is a Matlab keyword, and you can't use it as a variable name.
Let me give you some practical advice
When choosing a name for your variable, try to make it mnemonic so that you can easily remember what information it contains.
For instance, a variable named year would most likely hold information related to years.
>> year=2020
Also, it's best to avoid using names that have no clear meaning, such as x. This kind of name can make it hard to understand what information the variable holds.
For example, what information does the variable x contain? It's not easy to understand. It could be a phone number or a quantity.
>> x = 3283132412
Instead, you could give your variable a composed name, like "productcode".
>> productcode = 'abcdefg'
If you do, I'd suggest separating the words with an underscore (_) to make the name more readable at a glance.
>> product_code = 'abcdefg'
Alternatively, you could capitalize the first letter of the second word, as in productCode.
>> productCode = 'abcdefg'
But remember, these are just suggestions for good programming practices. Ultimately, you can choose any name you like for your variable.