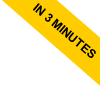
Cell array in Octave
In this lesson, I will explain how to create and use a cell array in Octave.
What is a cell array? A cell array is a special type of array that can contain other arrays within it. Each element of a cell array is called a cell, and each cell can contain an array of a different type (e.g. numeric, alphanumeric, etc.). However, each individual array within the cell array must contain elements of the same data type.
Creating an array of cells
To initialize a new array of cells, use the function cell(m,n), where m and n are the number of rows and columns of the cell array.
Here's a practical example.
Type cell(3,3) to create a 3x3 array of cells in the variable myVar.
>> myVar=cell(3,3)
myVar =
{
[1,1] = [ ](0x0)
[2,1] = [ ](0x0)
[3,1] = [ ](0x0)
[1,2] = [ ](0x0)
[2,2] = [ ](0x0)
[3,2] = [ ](0x0)
[1,3] = [ ](0x0)
[2,3] = [ ](0x0)
[3,3] = [ ](0x0)
}
When you create an array of cells, all the cells are empty.
Alternatively, you can also create an array of cells by specifying the data of the cells within curly braces.
>> A={"Math", "Latin", "Science"; 27, 30, 18}
A =
{
[1,1] = Math
[2,1] = 27
[1,2] = Latin
[2,2] = 30
[1,3] = Science
[2,3] = 18
}
Inserting arrays into a cell array
Once you have created the cell array, you can insert arrays into it.
For example, type myVar(1,1)=([1 2;3 4]) to store the array [1 2; 3 4] in cell (1,1).
>> myVar(1,1)=([1 2;3 4])
myVar =
{
[1,1] =
1 2
3 4
[2,1] = [ ](0x0)
[3,1] = [ ](0x0)
[1,2] = [ ](0x0)
[2,2] = [ ](0x0)
[3,2] = [ ](0x0)
[1,3] = [ ](0x0)
[2,3] = [ ](0x0)
[3,3] = [ ](0x0)
}
Then type myVar(1,3)=(['A' 'B' 'C' 'D']) to insert the array ['A' 'B' 'C'] into cell (1,3).
>> myVar(1,3)=(['A' 'B' 'C' 'D'])
myVar =
{
[1,1] =
1 2
3 4
[2,1] = [ ](0x0)
[3,1] = [ ](0x0)
[1,2] = [ ](0x0)
[2,2] = [ ](0x0)
[3,2] = [ ](0x0)
[1,3] = ABCD
[2,3] = [ ](0x0)
[3,3] = [ ](0x0)
}
Now the cell array consists of two arrays.
The two arrays have different dimensions and different data types. The first array is numeric while the second array is alphanumeric.
Note. When you insert arrays into cells, you do not have to follow a particular order. You can insert arrays into any cell. The other cells without arrays remain empty.
Reading the content of cells
You can access the content of each cell by indicating the coordinates (row, column) in round brackets.
For example, type myVar(1,3) to access the content of the cell (1,3).
>> myVar(1,3)
ans =
{
[1,1] = ABCD
}
You can also use the slicing technique to select multiple cells simultaneously.
For instance, type myVar(1:2,1:2) to select the first two rows and columns of the cell array.
>> myVar(1:2,1:2)
ans =
{
[1,1] =
1 2
3 4
[2,1] = [](0x0)
[1,2] = [](0x0)
[2,2] = [](0x0)
}
The latter command selects four cells.
Using this method, you can select multiple cells simultaneously.
How to display a cell array
To display the contents of a cell array, use the function celldisp()
For example, type celldisp(myVar) to display the cell array stored in the variable myVar.
>> celldisp(myVar)
myVar{1,1} =
1 2
3 4
myVar{2,1} = [ ](0x0)
myVar{3,1} = [ ](0x0)
myVar{1,2} = [ ](0x0)
myVar{2,2} = [ ](0x0)
myVar{3,2} = [ ](0x0)
myVar{1,3} = ABCD
myVar{2,3} = [ ](0x0)
myVar{3,3} = [ ](0x0)
In this lesson, you have learned how to use cell arrays in Octave.