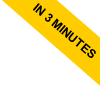
The structures in Octave
In this lesson, I will explain how to use structures in Octave with some practical examples.
What are structures? Structures, or arrays of structures, are similar to arrays. Unlike an array, a structure can store data of different types simultaneously, and the data is organized into fields. Each field has a name. A typical example of a structure is a phonebook.
How to create a structure
Create a structure to store a phonebook.
Name the structure "phonebook". The first field of the structure is the "name" of the person.
>> phonebook.name="Paul Red";
The second field of the structure is the person's "phone number".
>> phonebook.phonenumber=123456789;
If needed, a field's data can also be an array.
>> phonebook(1).phonenumber=[123456789, 987654321]
The third field of the structure is the person's address.
>> phonebook.address="Rome";
Alternatively, you can create the new structure by simultaneously adding all the fields using the struct() function.
>> phonebook = struct('name','Paul Red','phonenumber',123456789, 'address', 'Rome')
You have just created a structure (directory) with one record and three fields (name, phone, address).
Note. The fields have different data types. Two fields are alphanumeric (name and address) while one field is numeric (phonenumber).
If you wish, you can also create a new structure with multiple fields and data within it.
In this case, remember to write the field names only once and enclose the data of a field in curly braces.
C = struct('lesson',{'Math', 'Latin', 'Science'},'vote',{27,30,18})
How to display the fields of a structure
To display the list of fields in the structure, you can use the function fieldnames().
>> fieldnames(phonebook)
ans =
{
[1,1] = name
[2,1] = phonenumber
[3,1] = address
}
All records in the structure have the same number and names of fields.
In this case, the structure has three fields: name, phone, and address.
How to Add Data to a Structure
You can add additional records to the structure.
For example, to add a second record, use the name of the structure followed by the index number enclosed in parentheses
>> phonebook(2).name="Mario White";
>> phonebook(2).phonenumber="987654321";
Now the structure contains two records.
When adding a record to the structure, it is not necessary to fill in all fields. You can leave some fields blank.
For instance, in the second record of the structure, you did not document anything in the address field.
>> phonebook(2)
ans =
scalar structure containing the fields:
name = Mario White
phonenumber = 987654321
address = [](0x0)
Alternatively, you can add a new record by simultaneously entering all fields using the struct() function.
>> phonebook(3) = struct('name','Joseph Green','phonenumber',123456789, 'address', 'Milan')
How to access structure data
To access a data element within a structure, you need to write the structure name followed by the index enclosed in parentheses. After that, add a period and the name of the field you are interested in.
For example, to access the phone number of the first record in the structure, type phonebook(1).phonenumber.
>> phonebook(1).phonenumber
ans = 123456789
To read the phone number of the second record, type phonebook(2).phonenumber
>> phonebook(2).phonenumber
ans = 987654321
To display all data in the phone number field, type phonebook.phonenumber
>> phonebook.phonenumber
ans = 123456789
ans = 987654321
To display all data of a specific record, type phonebook(1)
>> phonebook(1)
ans =
scalar structure containing the fields:
name = Paul Red
phonenumber = 123456789 987654321
address = Rome
How to delete a field from a structure
To delete a field from a structure, you need to use the function rmfield()
For example, if you add a field to the structure, it now has four fields.
>> phonebook(2).test="test";
Now the struct has four fields.
>> fieldnames(phonebook)
ans =
{
[1,1] = name
[2,1] = phonenumber
[3,1] = address
[4,1] = test
}
To delete the "test" field, type rmfield(phonebook,"test")
>> phonebook=rmfield(phonebook,"test");
The "test" field has now been removed from the structure.
>> fieldnames(phonebook)
ans =
{
[1,1] = name
[2,1] = phonenumber
[3,1] = address
}
Now, you should now have all the necessary information to start working with structures in Octave.