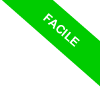
Comparison Operators in Python
In the realm of Python, comparison operators play a pivotal role, allowing us to juxtapose two values and yield a boolean outcome (`True` or `False`) contingent on the comparison's result.
Here's a succinct breakdown of the comparison operators at your disposal in Python:
Operator | Description | Example |
---|---|---|
== | Is equal to | a==b |
!= | Is not equal to | a!=b |
< | Is less than | a<b |
> | Is greater than | a>b |
<= | Is less than or equal to | a<=b |
>= | Is greater than or equal to | a>=b |
These tools are frequently employed within `if` statements and during the execution of `while` loops.
To better illustrate their application, consider this example.
Let's initialize two variables, "x" and "y", with the values:
x=2
y=3
Exploring the behavior of various comparison operators:
The '==' operator probes for equality between two values:
print(x==y)
This evaluates to False, given that 2 doesn't match 3.
False
The '!=' operator, on the other hand, investigates disparities:
print(x!=y)
This evaluates to True, underscoring the difference between 2 and 3.
True
The '>' operator assesses if the left-hand value surpasses the right:
print(x>y)
Given that 2 doesn't exceed 3, this returns False.
False
Conversely, the '<' operator determines if the left-hand value is overshadowed by the right:
print(x<y)
This rings true, as 2 is indeed less than 3.
True
The '>=' operator weighs whether the left-hand value is either greater than or on par with the right:
print(x>=y)
This returns False, as 2 neither exceeds nor equals 3.
False
The '<=' operator gauges if the left-hand value is either inferior to or matches the right:
print(x<=y)
Given that 2 is less than 3, this is validated as True.
True
A unique facet of Python is its ability to concatenate comparison operators, streamlining the comparison process. For instance, to ascertain if `x` lies exclusively between 0 and 10, you'd express it as 0<x<10.
print(0<x<10)
This construct confirms True if `x` is nestled between 0 and 10. Otherwise, it's False. In our scenario, with x=2, the statement 0<2<10 is undeniably true.
True
Armed with this insight, you're now well-equipped with a thorough understanding of Python's comparison operators, ready to harness their capabilities to the fullest.