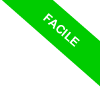
Python's Shift Operators
Python's shift operators play a pivotal role, enabling the manipulation of a number's bits by moving them either left or right.
Before delving deep into these operators, it's imperative to have a foundational understanding of numbers in their binary form.
Python boasts two primary shift operators:
Left Shift Operator (`<<`)
This operator adeptly shifts a number's bits to the left by a designated number of positions.
num << n
In this context, "num" represents the binary number under consideration, while "n" denotes the positions to shift leftward.
Consider the number five (5), represented in binary as "101".
x=5
Here, Python appends two zeros at the least significant end, morphing "101" into "10100". When we evaluate `y`:
y = x << 2
Python returns 20, the decimal counterpart of "10100".
print(y)
20
It's worth noting that a leftward shift by `n` positions effectively multiplies the number by 2n.
For instance, the operation `y = x << 2` mirrors the arithmetic `y = x·22`. Given x=5, y evaluates to 20.
Right Shift Operator (`>>`)
Conversely, this operator shifts a number's bits to the right by a specified count.
num << n
Here again, "num" signifies the binary number, and "n" is the shift magnitude.
To elucidate, let's assign 20 to variable "x".
x=20
In binary terms, 20 is represented as "10100".
Shifting its bits two positions to the right:
y = x >> 2
Python truncates the two least significant digits, converting "10100" to "101". On printing `y`:
print(y)
5
Python reveals the number 5, the binary equivalent of "101".
In wrapping up, a solid grasp of these two shift operators is instrumental in Python, especially when dealing with binary operations.