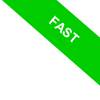
Python Operators
In Python, operators are distinct symbols designed to execute operations on variables and values. Their classification is based on their specific functionality:
Arithmetic Operators
These operators facilitate arithmetic computations between two operands, which can be either variables or values.
Operator | Description | Example |
---|---|---|
+ | Addition | a+b |
- | Subtraction | a-b |
* | Multiplication | a*b |
/ | Division | a/b |
% | Remainder | a%b |
** | Exponentiation | a**b |
// | Floor Division (returns the integer portion of the result) | a//b |
Comparison Operators
Such operators produce a boolean outcome (True or False) based on a comparison.
Operator | Description | Example |
---|---|---|
== | Equal to | a==b |
!= | Not equal to | a!=b |
< | Less than | a<b |
> | Greater than | a>b |
<= | Less than or equal to | a<=b |
>= | Greater than or equal to | a>=b |
Logical Operators
These are foundational in boolean logic, yielding a boolean result (True or False).
Operator | Description | Example |
---|---|---|
and | True if both expressions are true | a and b |
or | True if either expression is true | a or b |
not | True if the expression is false | not a |
Assignment Operators
They are employed to allocate a value to a variable.
Operator | Description | Example |
---|---|---|
= | Assign a value | a=1 |
+= | Increment and assign | a+=1 |
-= | Decrement and assign | a-=1 |
*= | Multiply and assign | a*=2 |
/= | Divide and assign | a/=2 |
%= | Assign remainder | a%=2 |
//= | Assign floor division | a//=2 |
**= | Assign exponentiation | a**=2 |
Operatori bitwise
These are the primary bitwise operators.
Operator | Description | Example |
---|---|---|
& | AND | a&b |
| | OR | a|b |
^ | XOR | a^b |
~ | NOT | ~a |
<< | Left shift | a<<1 |
>> | Right shift | a>>1 |
For a more comprehensive insight, consider reading our tutorial on left and right bit shift operations.
Identity Operators
They determine if two variables point to an identical object, returning a boolean value (True/False).
Operator | Description | Example |
---|---|---|
is | True if both variables point to the same object | a is b |
is not | True if the variables don't point to the same object | a is not b | a is not b |
Membership Operators
These operators ascertain if a value is part of an object, returning a boolean value (True/False).
Operator | Description | Example |
---|---|---|
in | True if the specified value exists within the object | a in b |
not in | True if the specified value doesn't exist within the object | a not in b | a is not b |
These operators are integral to Python programming. Each has its unique role, and their application varies based on the program's requirements.