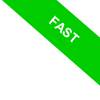
Binary File in MATLAB
In this tutorial, we'll explore how to write and read binary files using Matlab in an easy and friendly way.
So, what exactly are binary files? Well, they're files where data is stored as sequences of numbers in binary code, which is the language computers speak. You'll often find these files with a .bin extension. Since binary files are written in the computer's language, they're faster to process compared to text files, which contain information in human language.
How to Write a Binary File
Let's Write a Binary File.
To create a new binary file, simply use the fopen() function with the write attribute (w):
>> MyFile=fopen("test4.bin", "w");
It's the same command you'd use to open text files for writing. Easy, right?
Next, to write a record in binary code, go ahead and use the fwrite() function.
For example, just type fwrite(MyFile, [1:9]); to write a sequence of numbers from 1 to 9.
>> fwrite(MyFile,[1:9]);
Or, if you prefer, write a plain ol' string.
>> fwrite(MyFile,"prova")
Once you've finished writing, close the file using the fclose() function.
>> fclose(MyFile)
Congratulations! You've just created your first binary file.
By the way, the file is stored in the Matlab working folder.
A little trivia. When you write an alphanumeric string in a binary file, it's recorded as a sequence of Unicode/ASCII numeric codes. For instance, if you write fwrite(MyFile, "sample") in the file, the string "sample" is written as a sequence of numbers: 115 97 109 112 108 101, where 115=s, 97=a, 109=m, 112=p, 108=l, 101=e.
How to Open and Read a Binary File
To read a binary file, simply use the fopen() function with the read attribute (r)
MyFile=fopen("test4.bin", "r");
This way, you're opening the file for reading.
After that, use the fread() function to read the records in the binary file and save the data in the variable 'rec'.
>> rec = fread(MyFile)
Once you're done reading, don't forget to close the file using the fclose() function.
>> fclose(MyFile)
Now, if you take a look at the 'rec' variable, you'll see the data contained in the binary file.
>> rec
rec = 1
2
3
4
5
6
7
8
9
And there you have it! These simple steps will help you create or read any binary file with MATLAB. Happy coding!