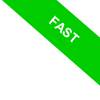
Creating and Reading Text Files in Matlab
In this easy-to-follow tutorial, I'll walk you through opening, creating, and reading text files in Matlab.
Creating a New File
To create a new text file, simply use the fopen() function.
Just put the name of the text file and the write access mode "w" (write) within the parentheses, like this:
>> MyFile=fopen("test2.txt", "w");
The MyFile variable is a convenient placeholder that you can use in the script instead of typing out the file name each time.
Now, let's write two records in the file using the fprintf() function.
>> fprintf(MyFile, "1 record \n");
>> fprintf(MyFile, "2 record \n");
Quick tip: The \n (newline) symbol at the end of each record tells Matlab to start a new line, creating a new record. If you don't include the \n symbol, the next fprint() or fput() instruction will continue writing on the same record.
Once you've finished writing, don't forget to close the file using the fclose() function.
>> fclose(MyFile);
Reading a File
Reading the contents of a text file is a piece of cake. Use the fopen() function and specify the file name and the "r" (read) attribute within the parentheses:
>> MyFile=fopen("test2.txt", "r");
The MyFile variable is a handy tool for performing operations on the file, and you can name it whatever you like.
Next, use the fgetl() function to read a record from the file.
>> fgetl(MyFile)
This function reads the contents of the first record.
ans = 1 record
To read the second record, just use the fgetl() function again.
>> fgetl(MyFile)
Now, the function reads the second record since the first record has already been read.
ans = 2 record
When the fgetl() function reaches the end of the file, it returns -1.
For example, if you type the fgetl() function for the third time,
>> fgetl(MyFile)
it returns -1, as there are only two records in the file, and both have already been read.
ans = -1
After completing the reading operations, remember to close the file with the fclose() function.
>> fclose(MyFile);
Reading a File with a Loop
When you need to read a file from start to finish, it's best to use a loop structure.
This way, you avoid typing fgetl() every time to read individual records.
MyFile=fopen("test4.txt", "r");
eof=0;
while eof==0
rec =fgetl(MyFile)
if (rec==-1) eof=1; endif
end
fclose(MyFile)
Appending Records to an Existing File
Want to add more records to an existing file without deleting the current content? No problem! Just open it in append mode.
For example, open the "test2.txt" file with the access mode 'a' (append) using the fopen() function:
>> MyFile=fopen("test2.txt", "a");
There are already two records in the file:
1 record
2 record
Now, add a new record using the fprintf() or fputs() function.
>> fprintf(MyFile, "3 record \n");
Tip: If you need to add even more records, keep using the fprint() function, following the example above.
Once you're done adding records, don't forget to close the file with the fclose() function.
>> fclose(MyFile);
Now, your file has three records.
1 record
2 record
3 record
Great job! Matlab added a new record without overwriting any existing records in the file.
File Access Modes
There are several handy file access modes to choose from:
r = opens the file for reading
w = opens a new file for writing
a = appends records to an existing file without erasing the current content
r+ = opens a file for both reading and writing
w+ = opens a new file for both reading and writing
a+ = opens a file for both reading and writing at the end of the file
You now have all the knowledge you need to start reading or writing text files using Matlab. Happy coding!