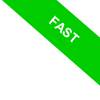
Creating a Delimited File in Matlab
In this easy-to-follow Matlab tutorial, I'm going to walk you through the process of creating a text file with numerical data separated by a delimiter.
Why should you care? Well, delimited numerical data files are super useful when you need to store and export data in ASCII format from one software to another. It's really quite handy!
Let's jump into a practical example together.
First, let's define a matrix.
>> M = [1 2 3; 4 5 6; 7 8 9 ];
Now, to save the matrix in a file with data separated by a delimiter, we'll use the dlmwrite() function.
dlmwrite('filename.txt',M,';')
The dlmwrite() function needs three bits of information:
- The name of the file to save the data
- The variable containing the data
- The character to use as a delimiter
This nifty little function saves the matrix in a text file on your computer's hard drive.
In our example, each record (row) in the file has numerical data separated by a semicolon ";".
Next, let's clear Matlab's memory using the clear function.
>> clear
Now, the M object is no longer in memory.
If you try to recall it, Matlab returns an error message.
>> M
Unrecognized function or variable 'M'.
To bring the M object back from the file and load it into memory, we'll use the dlmread() function.
>> X=dlmread('filename.txt', ';')
The dlmread() function also needs two bits of information:
- The name of the text file containing the data
- The character used as a delimiter
Matlab will then read the data from the text file and assign it to the array variable X.
Keep in mind, though, that to load the data, you need to specify the same delimiter character used to save the data in the file. In this case, the delimiter character is the semicolon.
Now, just type X on the command line and press enter.
>> X
X =
1 2 3
4 5 6
7 8 9
Voila! The variable X is now in Matlab's workspace and contains the same data as the matrix M.
With this method, you can save any numerical matrix in a file and load the data whenever you need it.
A quick note. In our example, we created a file using the semicolon ";" as a delimiter. But you can totally create text files separating the data with a different character, not just the semicolon. For instance, you can use a comma "," as a delimiter. To do that, simply modify the third parameter of the dlmwrite() function and the second parameter of the dlmread() function. Easy-peasy!