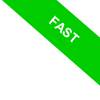
Do until loop in Matlab
In this lesson, I'll explain how to use the do until loop in Octave.
What's the do until loop, you ask? It's a looping structure that allows you to execute a block of code multiple times based on whether or not a certain condition has been met. It's similar to the while loop, except that in this case, the condition is checked at the end of each iteration.
So, how does it work?
The do until structure executes the block of code at least once.
In Matlab, there isn't a specific instruction for implementing the do until loop, but you can easily construct it using the while statement.
Here's a practical example:
cond=true;
x=1;
while cond
disp(x);
x=x+1;
if (x>9)
cond=false;
end
end
In this script, we assign the value of true to the variable cond and the numerical value of 1 to the variable x.
Then, we execute the block of instructions in the while loop that prints the value of x and increments it by one.
At the end of the block of code, we check whether the condition x>9 has been satisfied.
- If it hasn't, we execute a new iteration.
- If it has, we assign the value of false to the variable cond, which terminates the loop.
The output of this script is:
1
2
3
4
5
6
7
8
9
The script prints the values from 1 to 9, and the loop terminates when the variable x takes on the value of x = 10 because the condition x > 9 has been satisfied.
Note. Now, it's worth noting that in this example, we used the do until structure to implement a deterministic loop where we knew the total number of iterations from the start. You can also use the do until structure to implement indeterminate loops, but in these cases, you need to consider the risk of infinite loops.
The risk of an infinite loop arises when you use the do until loop to implement an indeterminate loop.
For example, consider this script that asks the user to guess a random number.
cond=true
while (cond)
numero=randi(100);
x = input("Enter a number between 1 and 100")
if not( x==number)
disp("You didn't guess right")
end
if (x==number)
disp("You guessed it")
cond=false;
end
end
This loop could iterate indefinitely (an infinite loop) if the user never guesses the random number.
To avoid the risk of an infinite loop, you can add a forced exit condition to the loop.
c=0;
cond=true;
while (cond)
number=randi(100);
x = input("Enter a number between 1 and 100")
if not( x==number)
disp("You didn't guess right")
end
if (x==number)
disp("You guessed it")
cond=false;
end
c=c+1
if (c==10)
disp("You have made too many attempts");
break;
end
end
In this modified script, we increment a counter variable (c) after each iteration of the loop.
After 10 iterations (user guesses), the script exits the loop even if the user hasn't guessed the random number yet.
This way, we avoid the risk of an infinite loop.