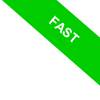
Matlab Loops
Let's talk about cyclic structures in Matlab.
What is a loop structure? A cyclic structure is a way to execute repetitive operations, and it's also known as a loop. Essentially, what you do is execute the same block of instructions multiple times, modifying the working variables each time. Each complete execution of the instruction block is called an iteration.
Now, Matlab has three main cyclic structures: the for loop, the while loop, and the do-until loop.
For Loop
Let's start with the for loop. This one executes a block of instructions for a predefined number of times. You do it like this:
for start:end
instruction block
end
As you can see, the for loop is a cycle that you can determine in advance.
You already know how many iterations you're going to do. Here's an example of a for loop:
for 1:9
disp(x);
end
This loop executes 9 iterations, printing a sequence of numbers from 1 to 9.
1
2
3
4
5
6
7
8
9
Now, if you want to execute an undetermined number of iterations, you can use the while or the do-until loop.
These are more powerful because they allow you to execute indefinite loops. The while loop executes a block of instructions repeatedly until a condition is met. It looks like this:
While loop
The while loop executes a block of instructions multiple times until a condition is satisfied.
while condition
block of instructions
end
As you can see, the while loop is indeterminate because you may not know how many iterations it will execute.
For example, an indeterminate loop could execute 10, 100 or even a billion iterations.
If the condition of the loop is not satisfied from the beginning, the while loop won't execute even a single iteration.
Note. One thing to keep in mind is that if the loop condition is always satisfied, you will end up with an infinite loop, which is one of the problems you absolutely want to avoid, as it consumes unnecessary system resources.
But you can use the while loop to execute both determinate and indeterminate loops.
Here's a practical example of a while loop:
x=1;
while x<10
disp(x);
x=x+1;
end
This loop prints out a sequence of numbers from 1 to 9.
1
2
3
4
5
6
7
8
9
Do until loop
Finally, let's talk about the do-until loop.
This one always executes at least one iteration, because the condition is checked at the end of each iteration.
In Matlab, there's no specific instruction for the do-until loop, but you can easily implement it using the while loop. Here's an example:
cond=true;
x=1;
while cond
disp(x);
x=x+1;
if (x>9)
cond=false;
end
end
This loop prints out a sequence of numbers from 1 to 9.
1
2
3
4
5
6
7
8
9
Well, these are the main cyclic structures in Matlab. Hopefully, this will help you understand how to execute repetitive operations more efficiently.