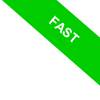
FOR loop in Matlab
Let's talk about the FOR loop in Matlab.
So what is a for loop? A FOR loop is a cyclic structure that executes the same block of code for a certain number of times, which we call iterations.
Here is the syntax for the FOR statement used in Matlab:
for variable = start:step:end
block of statements
end
Now, the first line of the FOR statement should indicate the loop counter variable and three parameters: the start, step, and end values.
- The start value is the initial value of the loop counter
- The step (or increment) is the amount by which the counter is incremented after each iteration
- The end value is the final value of the loop counter that determines the end of the loop.
The block of code is simply a sequence of instructions to be executed in each iteration of the loop.
The word "end" at the end of the block of statements indicates the end of the block of statements.
Note. The FOR loop is a "determined loop" because you already know how many iterations it will have to perform. If you want to create an "undetermined loop", you'll have to use the while or do-until instructions.
Now, let me give you a practical example.
Suppose we want to print the numbers from 1 to 9. We can easily do that using the FOR loop, like this.
for x=1:9
disp(x);
end
In this case, the loop counter variable is x, and the start and end values are 1 and 9, respectively.
We didn't specify the step, so Matlab uses a default increment of +1.
1
2
3
4
5
6
7
8
9
Now, if we want to print only the odd numbers from 1 to 9, we can use a step of 2, like this.
for x=1:2:9
disp(x);
end
The for function has three parameters 1:2:9, which indicate the start (1), the step (2), and the end (9) of the loop.
In this way, the script can print only the odd numbers from 1 to 9:
1
3
5
7
9
And finally, if we want to count down from 9 to 1, we can use a negative step, like this:
for x=9:-1:1
disp(x);
end
The for statement has three parameters 9:-1:1, which indicate the initial value (9), the step (-1), and the final value (1).
This script applies the negative step -1 after each iteration:
9
8
7
6
5
4
3
2
1
You can use the FOR loop to iterate over arrays or any other iterable object, too.
For example, in this script, the for x = v statement prints the elements of the array v = [1 2 3 4]
v = [1 2 3 4];
for x=v
disp(x);
end
The output result is the following:
1
2
3
4
I hope you found this explanation useful.