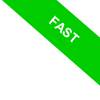
While Loops in MATLAB
In this lesson, I'd like to explain to you how to implement a while loop in Matlab.
So, what is a while loop? It's a repetitive structure, also known as a "loop," that allows you to execute a sequence of instructions multiple times as long as a condition is met. Each complete execution of the block of instructions is called an iteration.
The while loop checks a logical condition before executing the block of instructions.
while condition
block of instructions
end
Depending on the outcome of the check, the loop has two behaviors:
- If the initial condition is not satisfied from the beginning, the loop does not even complete one iteration.
- If the initial condition is satisfied, the loop executes the block of instructions. At the end of the execution, it starts over. The loop terminates when the initial condition is no longer satisfied.
Note. The while loop is an indefinite loop because you might not even know the number of iterations it will have to execute. It might not run at all, perform few or many iterations, and even never terminate (infinite loop).
Let me give you a practical example.
This code calculates the squares of numbers from 1 to 9 using a while loop.
x=1;
while x<10
disp(x^2);
x=x+1;
end
The loop performs nine "turns," or iterations.
In each iteration, it displays the square of the variable x and increments it by one.
The output is as follows:
1
4
9
16
25
36
49
64
81
In this case, the while loop executes a definite loop because the number of iterations is known from the beginning.
However, it's not always the case. A while loop can also perform an indefinite number of iterations.
It can even happen that the initial condition is always satisfied, leading to an infinite loop.
How to avoid the risk of an infinite loop?
To avoid this risk, I suggest you to insert a forced exit condition after a maximum number of iterations.
For example, this script generates a random number between 1 and 100. Then it asks the user to guess it.
number=randi(100);
x=0;
while x~=number
x = input("What number did I extract? ")
if (x~=number)
disp("Wrong!")
else
disp("You guessed it!")
break;
end
end
It's an example of an indefinite loop because you don't know how many times the user will try to guess the number.
The loop ends only when the user guesses the number.
Note. A rational user would make a maximum of 100 attempts to guess the number. However, if for some reason the user kept typing the same number... the loop would never end!
To avoid the problem of an infinite loop, introduce a maximum number of iterations in the while loop structure.
For example, add a counter variable t that increments after each iteration t = t + 1, i.e., after each attempt.
When the variable t equals 10, the loop terminates even if the user hasn't guessed yet.
number=randi(100);
x=0;
t=0;
while x~=number
x = input("What number did I extract?")
if (x~=number)
disp("Wrong!")
else
disp("You guessed it!")
break;
end
t=t+1
if (t==10)
disp("You made too many attempts!");
break;
end
end
This way, the user can make a maximum of ten attempts.
It's a simple trick to prevent the while loop from iterating infinitely.