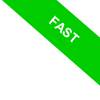
Do until loops in Octave
In this lesson, I'll show you how to create a do-until loop in Octave and explain what it is and how it works.
A do-until loop is another type of loop in Octave that allows you to repeat a block of instructions multiple times, also known as iterations. Unlike the for and while loops, the do-until loop is an indefinite loop because you don't know the number of iterations in advance.
The do-until loop first executes the block of instructions and then checks the condition.
- If the condition is not satisfied, it performs a new iteration.
- On the other hand, if the condition is satisfied, it exits the loop.
The syntax of a do-until loop is simple and consists of the keywords "do", "until", and the block of instructions.
do
block of instructions
until condition
Unlike the while loop, the do-until loop executes the block of instructions at least once, making it ideal for situations where you need to execute a specific instruction before testing a condition.
For instance, let's consider the following practical example of a do-until loop in Octave
- x=1;
- do
- disp(x);
- x=x+1;
- until x>9
In this program, the variable x is assigned the value of 1.
Then, a do-until loop is initiated, and the block of instructions is executed.
The block of instructions in this case comprises two instructions:
- the first instruction, "disp(x)", prints the value of the variable x
- the second instruction, "x=x+1" , increments the value of x by 1
Once the block of instructions is executed, the loop checks if the condition, "until x>9", is satisfied.
If the condition is not met, the loop starts a new iteration, executing the block of instructions again, and checking the condition again. If the condition is met, the loop exits.
In this particular case, the loop prints all values from 1 to 9, which means that it executed nine times before exiting the loop.
1
2
3
4
5
6
7
8
9
In conclusion, the do-until loop in Octave provides a powerful and flexible way to repeat a block of instructions multiple times until a specific condition is met.
The Risk of Infinite Loops and the Need for an Exit Condition
In the previous example, the do-until loop worked as a determined cycle because the maximum number of iterations was known. However, it's not always possible to predict the number of iterations in advance.
Undetermined loops are often used when the number of iterations is unknown.
For example, the following script prompts the user to guess a number:
- number=randi(100);
- do
- x = input("enter a number")
- if (x!=number)
- disp("you haven't guessed it")
- endif
- until x==number
Since this is an undetermined loop, it may continue to iterate indefinitely (an infinite loop), which can waste valuable computer resources.
To prevent infinite loops, it's important to include a forced exit condition in the loop. Here's an example:
- number=randi(100);
- c=0
- do
- x = input("enter a number")
- if (x!=number)
- disp("you haven't guessed it")
- c=c+1
- endif
- if (c==10)
- disp("you have made too many attempts")
- break;
- endif
- until x==number
In this example, a counter variable (c) is used to keep track of the number of iterations, and the loop is forced to exit after a maximum of 10 iterations.
This approach ensures that the loop will not run indefinitely and consume unnecessary resources.
By including a forced exit condition, you can avoid the risk of infinite loops and ensure that your code runs efficiently.