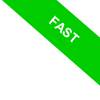
For loops in Octave
In this lesson, I'll explain how to create a loop with the for statement in Octave.
So, what is a for loop? It's a statement that allows you to cyclically execute a block of instructions for a specified number of times, with each execution of the block called an iteration.
The for statement is composed of an initial part "for start:step:stop", where you specify the start and end of the loop.
for variable = start:step:stop
block of instructions
end
The step (or increment) is the increase after each iteration. If not specified, it defaults to +1.
The block of instructions to be executed can consist of one or more statements.
The keyword "end" marks the end of the block of instructions.
Note that the for loop is a determined loop because you know the number of iterations it will perform from the start. For other types of undetermined loops, you can use the while and do-until statements.
Let's look at a practical example.
This script prints numbers from 1 to 9
for x=1:9
disp(x);
end
In this case, the range consists of two values, 1:9, which is the start (1) and the end (9).
The step is not specified, so it defaults to an increment of one (+1).
The output of the script is as follows:
1
2
3
4
5
6
7
8
9
You can also set a different increment.
For example, this script uses a step of +2 after each iteration:
for x=1:2:9
disp(x);
end
In this case, the range is composed of three values 1:2:9, that is, start (1), step (+2), end (9).
The script prints odd numbers from 1 to 9:
1
3
5
7
9
If you set a negative step, you can get a decrement instead of an increment.
For example, this script uses a decrement of -1 after each iteration:
for x=9:-1:1
disp(x);
end
In this case, the initial value (9) is larger than the final value (1) because the step is negative (-1).
The output is a countdown:
9
8
7
6
5
4
3
2
1
You can also use the for statement to iterate through the elements of an iterable object (e.g. array).
For instance, you can use the for loop to read the contents of an array
v = [1 2 3 4]
for x=v
disp(x);
end
This script iterates through the elements of the array from first to last and prints them on the screen.
1
2
3
4
Now you have a fairly complete overview of how for loops work.