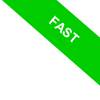
While loops in Octave
In this lesson, I will demonstrate how to construct a while loop in Octave.
So, what exactly is a while loop? It is a type of looping structure that enables you to execute a block of instructions repeatedly (i.e., through iterations) until a particular logical condition is met.
Before executing a block of instructions, the while loop verifies an initial logical condition.
The loop will continue until the initial condition is no longer satisfied, at which point the loop will terminate
while condition
block of instructions
endwhile
If the initial condition isn't satisfied from the beginning, the while loop won't even complete one iteration.
The while loop is considered an indefinite loop because you may not know the number of iterations beforehand.
Unlike other programming languages like Java or C, you don't have to write the code between curly braces in the while loop in some languages. However, it's essential to note that indentation, as in Python, isn't necessary either. To make the code more readable, I recommend writing the block of statements a little further to the right than the while statement.
For example, let's consider a practical use case where the loop calculates the square of numbers from 1 to 9
x=1;
while x<10
disp(x**2);
x=x+1;
endwhile
The output result is
1 4 9 16 25 36 49 64 81
In this case, the while loop completes a definite cycle because you know the number of iterations beforehand.
However, it's not always the case, and the while loop can also complete an indefinite number of iterations beforehand.
The Risk of an Infinite Loop
When writing a loop in a program, it's important to be mindful of the potential for an infinite loop.
An infinite loop occurs when the number of iterations is unknown, and the loop continues to run indefinitely. This can cause the program to become unresponsive and lead to system crashes.
To avoid this, it's always recommended to add a forced exit condition after a maximum number of iterations.
For instance, consider the following script that generates a random number between 1 and 100 and asks the user to guess it.
number=randi(100);
x=0;
while x!=number
x = input("What number did I draw? ")
if (x!=number)
disp("Wrong")
else
disp("You guessed it!")
break;
endif
endwhile
At first glance, it might seem like a simple loop that terminates when the user guesses the number.
However, if the user doesn't guess the number, the loop will run indefinitely, leading to an infinite loop.
To prevent this, we need to introduce a maximum number of iterations in the loop.
We can do this by adding a counter variable, t, that increments after each iteration.
When t reaches the maximum number of iterations, the loop will terminate even if the user hasn't guessed the number.
number=randi(100);
x=0;
t=0;
while x!=number
x = input("What number did I draw? ")
if (x!=number)
disp("Wrong")
else
disp("You guessed it!")
break;
endif
t=t+1
if (t==10)
disp("You made too many attempts.")
break;
endif
endwhile
By adding this condition, we can ensure that the loop terminates within a reasonable number of iterations, preventing the program from becoming unresponsive and avoiding potential system crashes.