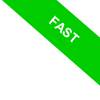
Loops in Octave
In this guide, I will show you how to create a loop using Octave.
But first, let's define what a loop is. It's a cyclic structure used for performing repetitive operations. By using different variables, a loop can execute the same operations multiple times. Each complete execution of the operations is referred to as an iteration.
There are several types of loops available, and we'll cover them all in this guide.
For loop
The for loop is a control flow statement that executes a block of instructions for a predetermined number of times. Its syntax is as follows:
for var=start:finish
block of instructions
end
Since you know the number of iterations beforehand, the for loop is considered a definite loop.
Here's a practical example of a for loop
for x=1:9
disp(x);
end
This script prints the numbers from 1 to 9
1
2
3
4
5
6
7
8
9
However, if you need to create indefinite loops, you can use the while or do-until statements.
While loop
The while loop executes a block of instructions as long as an initial condition is satisfied.
while condition
block of instructions
endwhile
It is an indefinite loop because you may not know the number of iterations in advance.
If the initial condition is not satisfied, the while loop does not execute even once.
Here's a practical example of a while loop
x=1;
while x<10
disp(x);
x=x+1;
endwhile
The output result is:
1
2
3
4
5
6
7
8
9
It's worth noting that in this case, the while loop behaves like a definite loop because the number of iterations is known in advance. In other cases, however, it behaves like an indefinite loop, depending on the condition to be satisfied. Therefore, a for loop can always be implemented using the while statement, but the reverse is not always true.
Do until loop
The do until loop is a type of loop that executes a block of statements until a certain condition is met.
The loop continues to execute until the final condition is satisfied.
do
block of instructions
until condition
This is an indefinite loop because you may not know the exact number of iterations the loop will go through.
In contrast to the while loop, the do until loop will always perform at least one iteration because the condition check is at the end of the block.
Here's an example of how a do until loop works:
x=1;
do
disp(x);
x=x+1;
until x>9
The output result of this code is:
1
2
3
4
5
6
7
8
9
These are the main looping structures in Octave.