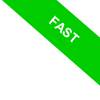
Calculating the determinant of a matrix in Python
Let’s dive into something that might seem a bit daunting but is actually quite fascinating: the determinant of a matrix. We’ll walk through how you can use NumPy to compute the determinant effortlessly.
What is a determinant? Don’t be intimidated by the term “determinant”—it’s simply a number that provides important information about square matrices. For instance, if the determinant of a matrix is zero, that matrix cannot be inverted. But first, a matrix is nothing more than a set of numbers arranged in rows and columns. Here’s an example of a 2x2 matrix: $$ A = \begin{bmatrix} 1 & 2 \\ 3 & 4 \end{bmatrix} $$ Does this look familiar? Probably! The determinant of a matrix like this is just a single number, and for a 2x2 matrix, it’s calculated like this: $$ \text{det}(A) = (1 \times 4) - (2 \times 3) = 4 - 6 = -2 $$ Pretty straightforward, right? But imagine having a much larger matrix, say 10x10. Doing the math by hand gets pretty tedious, doesn’t it? That’s where NumPy comes to the rescue!
How do you calculate the determinant with NumPy?
First, you’ll need to import the library and set up your matrix. Here’s a practical example.
import numpy as np
Next, define a 2x2 square matrix.
A = np.array([[1, 2], [3, 4]])
Remember, the determinant is only defined for square matrices—those with the same number of rows and columns.
Now you can calculate the matrix’s determinant using NumPy’s linalg.det() function, where “linalg” stands for "linear algebra".
det_A = np.linalg.det(A)
Finally, print the result.
print(f"The determinant of matrix A is: {det_A}")
The determinant of matrix A is: -2.0000000000000004
In this case, the result is very close to -2, but there’s a small difference due to the way computers handle numerical precision. Don’t worry about that “0.0000000000000004”—it’s just a rounding error!
An example with a larger matrix
Let’s try another example, this time with a 3x3 matrix. Curious to see how NumPy handles a larger matrix? Let’s find out!
B = np.array([[6, 1, 1],
[4, -2, 5],
[2, 8, 7]])
This time, we’re working with a 3x3 matrix, meaning 3 rows and 3 columns.
To calculate the determinant, you’ll again use the linalg.det() function.
det_B = np.linalg.det(B)
And print the result.
print(f"The determinant of matrix B is: {det_B}")
The determinant of matrix B is: -306.0
So, what does this tell us? This result means matrix B is invertible and carries some interesting properties.
The determinant gives you valuable insight into a matrix.
- If the determinant is zero, the matrix is “singular,” meaning it cannot be inverted.
- If the determinant is non-zero, you can invert the matrix, which is incredibly useful in many calculations, such as solving systems of linear equations.
Also, a non-zero determinant means the spaces spanned by the rows or columns of the matrix are independent—but I won’t go too deep into that here.
Once you get the hang of it, you’ll start to see determinants and matrices everywhere: in physics, engineering, economics… It’s a fundamental tool! And with NumPy, calculating them becomes a breeze.
I hope it’s now clear how to compute the determinant of a matrix using NumPy.