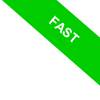
Creating matrices with NumPy in Python
In this lesson, we’ll learn how to create matrices in Python using the NumPy library.
What is a matrix? When I refer to matrices, I’m essentially talking about tables of numbers organized into rows and columns. For instance, here’s a matrix with two rows and three columns: $$ \begin{pmatrix} 1 & 2 & 3 \\ 4 & 5 & 6 \end{pmatrix} $$ You can perform a variety of operations on a matrix, like adding two matrices, multiplying them, calculating the determinant, finding the inverse, and so on.
In Python, a matrix can be thought of simply as a list of lists.
However, it’s much better to create them using NumPy because it’s not only easier but also significantly faster.
Additionally, NumPy offers numerous functions and methods that allow you to perform essential matrix operations with just a few lines of code.
Creating a matrix
Let’s start by creating a basic matrix with NumPy. First, load the module into memory:
import numpy as np
Next, create a 2x3 matrix with values arranged in 2 rows and 3 columns using the np.array() method:
matrix = np.array([[1, 2, 3], [4, 5, 6]])
So, what’s happening here? You’ve taken a list of lists in Python, `[[1, 2, 3], [4, 5, 6]]`, and passed it to the np.array() function.
Notice how the numbers in each row are separated by commas, and each row is enclosed in square brackets.
NumPy converts this list into a data structure known as an array, which holds the matrix.
If you run this code, you’ll get the following output:
[ [1 2 3]
[4 5 6] ]
And there you have it: a 2x3 matrix with two rows and three columns. Simple, right?
This object you just created corresponds to this matrix:
$$ \begin{pmatrix} 1 & 2 & 3 \\ 4 & 5 & 6 \end{pmatrix} $$
In the next sections, we’ll explore how to access elements within the matrix and perform some matrix operations.
Accessing elements
Now, let’s say you have this matrix `[[1, 2, 3], [4, 5, 6]]` stored in the variable "matrix," and you want to access a specific element.
For example, suppose you want to retrieve the value in the second row and second column:
You can do it like this: matrix[1, 1]
element = matrix[1, 1]
print(element)
Here, you’re instructing NumPy to access the element at position 1 (second row) and 1 (second column).
5
In this case, Python accesses and returns the value 5, which is located in the second row and second column of the matrix `[[1, 2, 3], [4, 5, 6]]`.
Remember, in Python, indices start at 0! So the first row has an index of zero, and likewise, the first column also has an index of zero.
Basic matrix operations
Once you’ve defined a matrix, you can perform a variety of mathematical operations on it.
For example, if you want to add a number to all the elements in the matrix `[[1, 2, 3], [4, 5, 6]]`, you can do so in one step:
new_matrix = matrix + 10
print(new_matrix)
This code adds 10 to every element in the matrix stored in the variable "matrix."
The result is stored in the variable "new_matrix," and the output is printed:
[ [11 12 13]
[14 15 16] ]
You can perform similar operations with subtraction (-), multiplication (*), or division (/).
These are all element-wise operations, meaning the operation is applied to elements at corresponding positions in the matrix.
This is incredibly useful because it eliminates the need to write nested loops to iterate over each element, saving you both time and effort.
Matrix multiplication
One of the most useful operations you can perform with matrices is row-column multiplication.
Here, we’re not talking about multiplying each element by a number, but rather performing actual matrix multiplication, as you would in linear algebra.
There’s a specific rule for doing this, and NumPy makes it easy using the dot() function.
For example, let’s create two 2x2 square matrices:
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
Now, calculate the row-column product of the two matrices using the dot() function:
C = np.dot(A, B)
print(C)
The result will be another matrix:
[[19 22]
[43 50]]
If you’re not familiar with matrix multiplication, imagine you’re multiplying the rows of the first matrix by the columns of the second.
Each element in the result is the sum of the products of corresponding numbers from the row and the column.
Here’s the step-by-step calculation of the row-column product: $$ \begin{pmatrix} 1 & 2 \\ 3 & 4 \end{pmatrix} \cdot \begin{pmatrix} 5 & 6 \\ 7 & 8 \end{pmatrix} = \begin{pmatrix} 1 \cdot 5 + 2 \cdot 7 & 1 \cdot 6 + 2 \cdot 8 \\ 3 \cdot 5 + 4 \cdot 7 & 3 \cdot 6 + 4 \cdot 8 \end{pmatrix} = \begin{pmatrix} 19 & 22 \\ 43 & 50 \end{pmatrix} $$
Other useful operations
NumPy is incredibly versatile when it comes to matrix operations. It allows you to perform a wide range of tasks with ease. Here are a few examples:
- Transposing a matrix: Swap rows with columns, and vice versa.
transpose = np.transpose(matrix)
print(transpose)[[1 4]
[2 5]
[3 6]] - Determinant of a matrix: For square matrices, you can compute the determinant, an important value in linear algebra. Keep in mind that Python calculations may include small rounding errors.
det = np.linalg.det(A)
print(det)-2.0000000000000004
- Inverse of a matrix: You can also find the inverse of a square matrix, which is useful for solving linear systems.
inverse = np.linalg.inv(A)
print(inverse)[[-2. 1. ]
[ 1.5 -0.5]]
In conclusion, matrices might seem intimidating at first, but once you understand how they work, you’ll realize they ’re powerful and easy to use.
For many people, matrices might seem like a complex and dry topic, but trust me, once you grasp the basics, you can accomplish some amazing things with them.
Why use the NumPy library?
NumPy simplifies matrix calculations, making operations that would normally be time-consuming and complex much more manageable in Python.
The beauty of NumPy is that it allows you to perform even complex matrix operations with just a few lines of code. That’s a huge advantage!
This is why NumPy is one of the most popular Python libraries among data scientists.