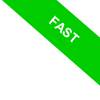
NumPy
NumPy is a Python library that simplifies working with numbers and tabular data, treating them as tables or matrices.
What is this module for? Think of NumPy as a library that lets you create arrays, perform matrix calculations, and generate faster, more powerful lists. It’s an essential tool in Python, especially when dealing with large datasets.
To fully understand the power of this library, consider the following scenario.
You have a list of numbers and want to add 5 to each one. Using standard Python lists, you would need to write a “for” loop to add 5 to every element.
With NumPy, however, you can achieve the same result in just one line, without any loops. Here's an example:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = a + 5 # b becomes [6, 7, 8, 9, 10]
As you can see, it’s much more straightforward.
Moreover, NumPy is written in C and Fortran under the hood, which means operations on arrays are significantly faster than using standard Python lists.
NumPy is the backbone of many other scientific and machine learning libraries, such as Pandas (for data analysis), SciPy (for advanced scientific computations), Matplotlib (for plotting), and TensorFlow (for machine learning). So, learning NumPy sets you up to explore a wide range of powerful tools in Python!
But that’s just the beginning. NumPy enables you to do much more.
What you can do with NumPy
Here are a few useful things you can do with NumPy:
- Create number arrays
Want to create a list of numbers from 0 to 9? Easy!a = np.arange(10) # a becomes [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
- Perform complex mathematical calculations
You can multiply, divide, find averages, or calculate square roots of entire arrays of numbers in one go.a = np.array([1, 4, 9, 16])
roots = np.sqrt(a) # roots becomes [1, 2, 3, 4] - Work with multidimensional arrays
NumPy allows you to work not just with lists, but also with tables (or 2D arrays) like matrices and tensors. It’s like working with spreadsheets, but much more powerful.matrix = np.array([[1, 2], [3, 4]])
- Matrix operations
If you've ever worked with matrices in linear algebra, NumPy makes matrix operations incredibly easy. You can multiply matrices, transpose them, or calculate determinants and inverses without any hassle.a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6], [7, 8]])
result = np.dot(a, b) # Multiplies matrices a and b - Generate random number arrays
Need random numbers? NumPy has a `random` module that lets you generate arrays with randomly distributed numbers. For example, here’s how to create a 3x3 matrix of random numbers between 0 and 1:random_numbers = np.random.rand(3, 3)
- Statistical functions
NumPy is ideal for performing statistical calculations. You can quickly compute the mean, standard deviation, maximum, and minimum in just a few steps.data = np.array([10, 20, 30, 40, 50])
mean = np.mean(data) # Calculates the mean
std_dev = np.std(data) # Calculates the standard deviation - Array slicing and masking
NumPy makes it easy to work with portions of arrays using slicing. You can also filter data based on conditions, selecting only elements that meet certain criteria. For instance, select only the second through fourth numbers:a = np.array([1, 2, 3, 4, 5, 6])
Here’s an example of masking, which returns only values greater than 3 from an array:
sub_array = a[1:4] # output: [2, 3, 4]a = np.array([1, 2, 3, 4, 5, 6])
mask = a[a > 3] # output: [4, 5, 6] - Reshaping arrays
A fantastic feature of NumPy is the ability to reshape arrays, transforming a flat (1D) array into a matrix or an array of different dimensions, as long as the number of elements remains the same.a = np.arange(12) # Creates an array with numbers from 0 to 11
a_reshape = a.reshape(3, 4) # Reshapes the array into a 3x4 matrix - Numerical derivatives and integrals
If you're working with complex mathematical functions, you can use NumPy to approximate derivatives and integrals. While more specialized libraries like SciPy are commonly used, NumPy already has functions for quick estimates. Here’s an example of an approximate derivative:x = np.linspace(0, 10, 100) # Creates 100 values between 0 and 10
y = np.sin(x) # Calculates the sine of each x value
dy_dx = np.gradient(y, x) # Approximates the derivative - Universal functions (ufunc)
Ufuncs are functions that apply element-wise operations to arrays. You can use them for operations like sine, cosine, logarithms, exponentials, and more.a = np.array([0, np.pi/2, np.pi])
sine = np.sin(a) # Applies the sine function to each element in the array
In summary, NumPy is your go-to choice for fast, efficient handling of numerical data without writing a lot of extra code.
It’s especially useful for mathematical calculations, data analysis, and working with matrices, graphs, and much more.
If you’re planning to dive into data analysis or machine learning, mastering NumPy is an essential first step.
How to install NumPy in Python
Installing NumPy in Python is straightforward and can be done directly from your command line or terminal.
If you already have Python installed, you probably also have pip, Python’s package manager, installed.
To install NumPy, simply enter this command in your terminal:
pip install numpy
This command will download and install the latest version of NumPy.
If you’re using Anaconda, a popular Python distribution for data science and machine learning, you can install NumPy using conda. Open the Anaconda terminal or command prompt and type:
conda install numpy
This will install NumPy within your Anaconda environment.
After installing NumPy, you can check whether the installation was successful by opening a Python interface (like a Python console, Jupyter Notebook, or a `.py` file) and typing:
import numpy as np
print(np.__version__)
1.21.5
If no errors occur and the terminal returns the NumPy version, the installation was successful!
If the installation fails, here are a few things to check:
- Is your Python version compatible with NumPy?
Ensure that your Python version is supported by NumPy, typically Python 3.x. - Is pip installed on your system?
If you get an error saying pip is not recognized, you might need to add Python and pip to your system’s PATH environment variable. Alternatively, try using `python -m pip install numpy`.
Once the installation is complete, you’re ready to start using NumPy in your Python code by importing the library.
How to use the NumPy library
After installing NumPy, there are two main ways to import it, depending on your needs:
A] Import the entire library
You can import NumPy using the import statement. This is the most common method and gives you access to all of NumPy’s features.
import numpy as np
This command imports the entire NumPy library and, by convention, assigns it the alias `np` for easier access to its functions.
This means that whenever you use a NumPy function, you’ll need to prefix it with `np`.
a = np.array([1, 2, 3])
b = np.sin(a)
print(b)
This code calculates the sine of all elements in the array
[0.84147098 0.90929743 0.14112001]
This import method is recommended if you plan to use many of NumPy’s functions.
B] Import specific functions only
If you only need a few specific functions from NumPy, you can import just those functions or classes using the from import statement. This approach saves memory and can make your code more readable. Here’s how:
from numpy import array, sin
In this case, you can use the imported functions directly without needing to prefix them with `np`.
For example, you can write the following code:
from numpy import array, sin
a = array([1, 2, 3])
b = sin(a)
print(b)
[0.84147098 0.90929743 0.14112001]
This approach is useful when you only need a few functions, such as in smaller scripts, or to avoid overwriting functions with the same name from other libraries.
Whichever method you choose, you’ll be ready to leverage NumPy’s powerful features in your code!
Remember, you only need to import the NumPy library (or any other library) once at the beginning of your script, or when you start a terminal session. Once imported, you can use NumPy throughout your script or session without importing it again.
Now you have everything you need to get started with NumPy.