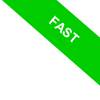
Matrices and Vectors in Scilab
In the Scilab environment, you're presented with an extensive suite of tools designed specifically for matrix and vector computations, as well as their manipulation.
Creating Matrices and Vectors
To craft a vector, simply enclose its elements within square brackets.
For a row vector, delineate the elements with commas.
v = [1, 2, 3]
This arrangement positions the elements horizontally in a single row.
v =
1. 2. 3.
Conversely, to establish a column vector, elements should be separated with semicolons.
v = [1; 2; 3]
In this configuration, the elements stack vertically in a singular column.
v =
1.
2.
3.
Another approach to formulating a column vector is by transposing a row vector. Define your row vector and transpose it by appending an apostrophe at its conclusion.
v = [1, 2, 3]'
Upon doing so, Scilab rotates the row vector 90° clockwise, rendering it a column vector.
v =
1.
2.
3.
When it comes to matrices, elements within the same row are separated by commas, while different rows are demarcated with semicolons.
For example, to construct a 2x2 matrix with two rows and columns under the variable "M"
M = [1, 2; 3, 4]
Scilab systematically organizes these four elements into a two-dimensional framework.
M =
1. 2.
3. 4.
Vector and Matrix Operations
Once vectors or matrices are defined, they become powerful tools for a range of matrix and vector computations.
Addition and Subtraction
Matrices of identical dimensions can be added or subtracted. This means they should have an equal number of elements arranged similarly.
Consider two 2x2 matrices.
A = [1, 2; 3, 4];
B = [2, 2; 2, 2];
Now, add the two matrices.
C = A + B;
This yields another 2x2 matrix with the sum of the corresponding elements.
C =
3. 4.
5. 6.
It's essential to note that matrices can only be combined if they share the same number of rows and columns.
In a similar vein, vectors can be added if they have an equal number of elements and share the same orientation, either as row or column vectors.
Multiplication
Multiplication offers two distinct methods:
- Element-wise Multiplication
This approach calculates the product of matching elements between two vectors or matrices of identical size. The ".*" operator facilitates this:
C = A.*B
Using the matrices A and B
A = [1, 2; 3, 4]
Let's compute the element-wise multiplication.
B = [2, 0; 1, 3]
C = A.*B
Resulting in:C = [2, 0;
It's imperative that A and B are of matching size for this operation. A mismatch will trigger an error in Scilab.
3, 12] - Matrix Multiplication (Row by Column)
This method involves the row-by-column product. Here, matrix C results from the summation of products from A's rows and B's columns:C = A*B
For this operation, the column count of the first matrix should align with the row count of the second. Using the matrices:A = [1, 2; 3, 4];
Let's compute the matrix multiplication.
B = [2, 0; 1, 3];C=A*B
The result is
C =
4. 6.
10. 12.
Division
Division also presents two operational methods:
- Element-wise Division
This technique produces a matrix where each quotient matrix element results from dividing A's corresponding elements by B's. It's done using the "./" operator.
C = A./B
For example, consider the matrices A and B, both 2x2
A = [9, 6; 8, 6];
Then compute the element-wise division
B = [3, 2; 4,3];C = A./B
The element-wise division isC =
For this operation, A and B must be of matching size. Additionally, ensure B has no zero elements, as this would lead to an undefined mathematical operation.
3. 3.
2. 2. - Matrix Division
The expression C = A/B is a solution to the linear equation system A*X = B, where X is our sought-after matrix. This is achieved with the "/" operator.
C = A/B
For this, the column count of the first matrix should align with the row count of the second. For instance, consider these 2x2 matrices:
A = [9, 6; 8, 6];
Then compute the division between the two matrices
B = [3, 2; 4,3];
C = A/B
Resulting inC =
An important takeaway: the same outcome can be derived by multiplying matrix A by matrix B's inverse. Thus, C = A/B is synonymous with C = A*inv(B), where inv(B) represents the inverse of matrix B.
3. 0.
0. 2.
Useful Functions for Matrix and Vector Calculations
Scilab boasts an array of functions tailored for matrix and vector operations.
Let's delve into some of the most pivotal ones:
- Transpose
Transposing a matrix or vector in Scilab is straightforward. Simply append an apostrophe to the variable name. For instance, using `A'` yields the transpose of matrix A.
A'
- Determinant
To determine the determinant of a square matrix A, the `det()` function is your go-to.det(A)
- Inverse Matrix
Calculating the inverse of a matrix? The `inv()` function has you covered.
invA)
- Eigenvalues and Eigenvectors
The `spec()` function retrieves the eigenvalues of a matrix.
spec(A)
For a comprehensive output including both eigenvectors (V) and a diagonal matrix (D) of the eigenvalues, use:
[V, D] = spec(A)
Matrix and Vector Manipulation
To wrap things up, here are some handy operations for managing matrices and vectors in Scilab:
Subvector and Submatrix Extraction
Extracting a submatrix is seamless in Scilab. For instance, given matrix A:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
A 2x2 matrix can be extracted as
subA = A(1:2, 2:3)
This command selects the submatrix from the first two rows and the last two columns.
subA =
2. 3.
5. 6.
Resizing
Reshaping matrices in Scilab is intuitive. Consider the 2x3 matrix:
A = [1, 2, 3; 4, 5, 6];
This matrix has two rows and three columns.
A =
1. 2. 3.
4. 5. 6.
This matrix can be resized to a 3x2 dimension using:
B = matrix(A, 3, 2)
The result rearranges the elements of matrix A into three rows and two columns.
B =
1. 5.
4. 3.
2. 6.
Concatenation
Scilab facilitates the concatenation of matrices or vectors. Given two matrices:
A = [1, 2; 3, 4];
B = [2, 0; 1, 3];
For horizontal concatenation, type C = [A, B]
C = [A, B]
The outcome is a 2x4 matrix
C =
1. 2. 2. 0.
3. 4. 1. 3.
Alternatively, for vertical concatenation, type C = [A; B]
C = [A; B]
The outcome is a 4x2 matrix
C =
1. 2.
3. 4.
2. 0.
1. 3.
In the domain of matrix and vector operations, these tools are paramount for a wide range of engineering and scientific tasks.
In essence, Scilab's robust library ensures that any matrix or vector computation is within your grasp.