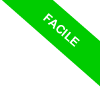
Assert Statement in Python
Today, we'll discuss a very useful and often underestimated tool in Python: the `assert` statement.
In Python, `assert` is a statement used to verify if a condition is true. The syntax of `assert` is straightforward:
assert condition, error_message
This statement takes two arguments:
- The `condition` is the expression you want to check.
- The `error_message` is optional but highly recommended. It is the message that will be displayed if the assertion fails.
If the condition is false, the program execution is halted, and an `AssertionError` exception is raised.
In essence, `assert` allows you to insert checkpoints in your code to ensure certain conditions are met during execution.
Why use assert? Utilizing `assert` is particularly beneficial during development and debugging. It helps verify that the assumptions made by the programmer are correct. Remember, `assert` should never be used in a production environment because it can be disabled in some Python settings.
Let's examine some practical examples to understand how to use `assert` in code.
Suppose we have a function that calculates the square root of a number. We want to ensure that the input is a positive number.
import math
def square_root(x):
assert x >= 0, "Input must be a non-negative number"
return math.sqrt(x)
If you call the function with a positive number, it returns the square root of that number:
print(square_root(9))
3.0
If you call it with a negative number, an AssertionError is raised:
print(square_root(-4))
AssertionError: Input must be a non-negative number
Let's look at another example.
We want to ensure that an attribute always remains within a certain range.
class Temperature:
def __init__(self, degrees):
self.set_degrees(degrees)
def set_degrees(self, degrees):
assert -273.15 <= degrees <= 1000, "Invalid temperature: must be between -273.15 and 1000 degrees Celsius"
self._degrees = degrees
def get_degrees(self):
return self._degrees
Now, create an instance of the class with 25 as the parameter.
t = Temperature(25)
When you access the object, it displays the value you assigned:
print(t.get_degrees())
25
Now try to change the value of degrees to -300:
t.set_degrees(-300)
In this case, an AssertionError is raised because the value is out of range. It is below absolute zero (-273.15).
AssertionError: Invalid temperature: must be between -273.15 and 1000 degrees Celsius
The same would happen if you provided a value greater than 1000.
When Not to Use assert
The assert statement should be used solely for internal checks during development and debugging.
It should never be used in a production environment because `assert` can be disabled by running Python with the `-O` (optimize) option, which would bypass the checks during execution.
Using the -O option turns off Python's debug mode (__debug__), which is enabled by default.
Therefore, it is not reliable for handling critical conditions that must always be verified.
For example, user inputs should always be verified with explicit conditions and exception handling, never with the 'assert' statement.
Additionally, the AssertionError exception is quite broad and doesn't provide specific details about the problem.
I hope you found this guide helpful.