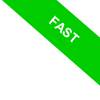
The Difference Between Errors and Exceptions
In Python programming you'll frequently encounter two significant events: errors and exceptions. Despite their similarities - both represent unexpected or non-conforming situations - they are distinct entities with notable differences.
Errors: Pre-Execution Hurdles
Before a Python program takes its first breath, it could stumble upon errors. These are often syntax errors, little tripwires that impede the program from striding ahead into execution.
Consider you missed closing a parenthesis or attempted to employ an undefined variable. In these instances, Python dons the referee's whistle, flags an error, and halts the march of your program.
Let's dissect an example:
print("Hello, World!"
Running the script, Python promptly slams the brakes and flashes the dreaded "Syntax Error" sign:
SyntaxError: missing closing parenthesis
Your hands are tied here. Until you fix the syntax error lurking in your code, your program stays grounded.
These errors are spotted and flagged at the starting line, during the transformation of your code into bytecode. Technically, your program's execution isn't interrupted; it's prevented from firing up due to the error.
Exceptions: Mid-Execution Missteps
Exceptions are unexpected situations encountered while your program is off to the races, running despite the code being syntactically flawless. They act as red flags, compelling the program to a screeching halt.
Suppose your program attempts a division by zero or embarks on a fruitless search for a phantom file. In such scenarios, Python steps in, brandishing an exception.
Here's how an exception manifests in practice
print(5 / 0)
The command above, though syntactically accurate, tempts Python to raise the ZeroDivisionError exception, as it's trying to pull off a mathematically impossible operation.
# ZeroDivisionError: division by zero
Here, Python runs the program because the issue doesn't rear its head during the bytecode compilation stage. Instead, it crops up during the race - while the program is in execution.
Managing Exceptions: Grabbing the Reins
Unlike errors, exceptions don't spell the end of your program's journey. You can rein them in without putting a full stop to your program.
Python empowers you to handle these exceptions using the try and except blocks.
- try:
- print(5/0)
- except ZeroDivisionError:
- print("Hai provato a dividere per zero!")
Implementing try and except blocks enables your program to navigate around the pothole of an exception and continue its run.
On the contrary, errors aren't so forgiving. They refuse to be managed in this manner, demanding rectification in the code before your program can even take off.