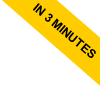
Try-Except Statement in Python
In the world of Python, a godsend comes in the form of the 'try-except' composite statement. This powerful tool gives you the ability to expertly handle exceptions, which are those pesky errors that sneak up on you during program execution.
- try:
- #code block that we're putting to the test
- except:
- #code block that springs into action if an exception rears its ugly head
So, here's how it works: Python initially executes the block of code that trails the 'try' keyword.
If an exception comes along, the program swiftly intercepts it and executes the block right after the 'except' clause.
So, you may be wondering why we need this. Well, exceptions aren't your regular syntax errors. They can show up unexpectedly depending on the data and conditions. Case in point: a division by zero, or an attempt to open a phantom file. When these problems occur, they can bring the execution of your program to a standstill and cause a crash. But the try-except construct has got you covered and helps you prevent this.
Here's a hands-on example.
Consider the following script.
- x=10
- y=0
- z=x/y
- print(z)
Although this script is free from syntax errors, the program grinds to a halt when you run it because it attempts a division by zero.
Traceback (most recent call last):
File "/home/main.py", line 3, in <module>
z=x/y
ZeroDivisionError: division by zero
Frustrating, right? Well, you can sidestep this issue by rewriting the script using the try-except statement.
- try:
- x=10
- y=0
- z=x/y
- print(z)
- except ZeroDivisionError:
- print("Hold up! You can't divide by zero!")
Upon running the program now, Python raises its red flag, the ZeroDivisionError exception.
This exception is snagged by the 'except ZeroDivisionError' construct, which in turn displays a cautionary message.
Hold up! You can't divide by zero!
This time, your program stays on its feet and execution resumes as planned.
However, do bear in mind that the 'except ZeroDivisionError' clause is a specialist—it only deals with the 'ZeroDivisionError' exception. If any other exception tries to gatecrash during execution, it will still crash the party.
Is it possible to catch multiple exceptions?
Absolutely! To tackle multiple exceptions, you can simply include more 'except' clauses in the structure.
If two or more clauses handle the same exception, only the first one will be executed.
Here's a practical example.
- try:
- x=10
- y=2
- z=x/y
- print(z)
- except ZeroDivisionError:
- print("Hold up! You can't divide by zero!")
- except ValueError:
- print("Enter a digit, please. Like 1, 2, 3, etc.")
- except:
- print("An uninvited exception just showed up!")
In this setup, we've got three 'except' clauses on the watch.
- Our first guard, 'except ZeroDivisionError', jumps into action only when a division by zero tries to break in.
- The second watchman, 'except ValueError', steps up if the values are non-numeric.
- The third protector, simply 'except', doesn't look for a specific exception. Its job is to catch anything suspicious that might occur.
A word of caution: it's not advisable to use an 'except' clause without specifying an exception type. Yes, the program will still run, but it can leave you in the dark about what's truly malfunctioning in your program.
Sometimes, you may want to manage multiple exceptions within the same clause.
In these instances, you need to call out a tuple of exceptions after the 'except' clause.
- try:
- x=10
- y=2
- z=x/y
- print(z)
- except (ZeroDivisionError, ValueError):
- print("The value is either zero or it's not a number at all!")
Moreover, the try-except structure graciously accommodates the 'else' and 'finally' clauses as well.
The 'else' block swings into action only if the code in the 'try' block runs without raising an exception.
The 'finally' block, a real trooper, always executes, no matter whether an exception gatecrashes the party or not.
Here's a concrete example:
- try:
- x = 1 / 1
- except ZeroDivisionError:
- print("Hold up! You can't divide by zero!")
- else:
- print("Hurray! The division was a success!")
- finally:
- print("End of calculation")
In this case, the code in the 'try' block doesn't stir up any trouble, so the 'else' block takes its turn and proudly announces "Hurray! The division was a success!".
Then, come what may, Python runs the 'finally' block, declaring "End of calculation".
Hurray! The division was a success!
End of calculation
Now, if you dare to write x=1/0 in the third line of the code, the 'try' block stirs up a 'ZeroDivisionError' exception.
- try:
- x = 1 / 0
- except ZeroDivisionError:
- print("Hold up! You can't divide by zero!")
- else:
- print("Hurray! The division was a success!")
- finally:
- print("End of calculation")
This time around, the 'except' block gets its turn, and the 'else' block sits this one out.
But as always, the 'finally' block springs into action no matter what.
Hold up! You can't divide by zero!
End of calculation
By using this method, you're equipping yourself to deftly handle an array of situations and events that could emerge during computation.