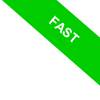
The 'args' Attribute in Python Exceptions
In Python, the args attribute is found in all exceptions and contains all the arguments passed when the exception instance is created.
These arguments are stored in the args attribute as a tuple.
For example, in this code, we try to divide a number by zero.
try:
x=1
y=0
z=x/y
print(z)
except ZeroDivisionError as e:
print(e.args)
The ZeroDivisionError exception is caught and assigned to the variable "e".
If we examine the e.args attribute, Python returns a tuple with some arguments:
('division by zero',)
The same applies to custom exceptions.
In this simple example, we raise an exception with two arguments, and Python stores them in the args attribute.
class MyException(ZeroDivisionError):
pass
try:
raise MyException("Division by zero",2024)
except MyException as e:
print(e.args)
('Division by zero', 2024)
This behavior is inherited from the BaseException class and remains consistent even when the constructor (__init__) of the custom exception class is overridden.
In this example, we modify the constructor of the custom exception and create an instance.
The args attribute will contain the arguments passed during the instantiation of the exception.
class MyException(ZeroDivisionError):
def __init__(self, msg, year):
self.msg = msg
self.year = year
ex = MyException("Division by zero", 2024)
print(ex.args)
('Division by zero', 2024)
So, even when we override the constructor to add a custom attribute (msg), the arguments are still included in args.