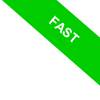
Raise Statement in Python
In Python, the raise statement allows you to manually trigger an exception.
raise ExceptionType("Error message")
Here, `ExceptionType` is the type of exception you want to raise (e.g., `ValueError`, `TypeError`, `RuntimeError`, etc.).
The argument in parentheses is optional and is a string that describes the error. This message will be displayed when the exception is caught.
What is an exception? An exception is an event that occurs during the execution of a program and disrupts the normal flow of instructions. In Python, exceptions are used to handle errors that may occur while running the code. When an exception is triggered, the program immediately halts the current function's execution and passes control to the appropriate exception handler.
Why raise an exception manually?
There are various reasons for doing this, such as when you want to halt a function's execution due to invalid input or an unmanageable condition.
Let's look at some practical examples to clarify how to use the `raise` statement.
Imagine you have a function that calculates the reciprocal of a number. If the input number is zero, division by zero will raise an exception. We can use `raise` to handle this situation explicitly.
def calculate_reciprocal(number):
if number == 0:
raise ValueError("Cannot calculate the reciprocal of zero.")
return 1 / number
try:
result = calculate_reciprocal(0)
except ValueError as e:
print(f"Error: {e}")
In this example, if `number` is zero, we raise a `ValueError` with a clear message. In the `try` block, we catch the exception and print the error message.
Since we call the function with zero as the argument, the program's output is:
Cannot calculate the reciprocal of zero.
You can also create your own custom exceptions by defining a new class that inherits from `Exception`.
class NegativeNumberError(Exception):
pass
def calculate_square_root(number):
if number < 0:
raise NegativeNumberError("Cannot calculate the square root of a negative number.")
return number ** 0.5
try:
result = calculate_square_root(-4)
except NegativeNumberError as e:
print(f"Error: {e}")
In this example, we defined a custom exception `NegativeNumberError` and used it to raise an error when a negative number is passed to the `calculate_square_root` function.
We then call the function with -4 as the argument.
Since it's a negative number, the exception is raised, and the program responds with:
Error: Cannot calculate the square root of a negative number.
In general, the raise statement is very useful when you need to signal an error that cannot be handled within the current function. It allows you to stop a function's execution due to invalid input or an unusual condition. It's also useful when developing a module or library and you want to provide clear error messages to users of your code.
Always remember to handle exceptions appropriately using try-except blocks, and to provide helpful error messages that can assist in diagnosing and resolving issues.
Raise From
There are times when, while handling an exception, it can be useful to raise another one.
This can be necessary, for example, when you need to provide additional context or when one exception is the direct result of another. This is where raise from becomes relevant.
Consider a function that converts a string to an integer.
def convert_to_int(s):
try:
return int(s)
except ValueError as e:
raise ValueError(f"Conversion error: '{s}' is not a valid number") from e
def main():
user_input = "abc"
try:
number = convert_to_int(user_input)
print(f"The number is: {number}")
except ValueError as e:
print(e)
main()
In this code, the function convert_to_int() attempts to convert the string `s` into an integer using int(s).
If the conversion fails, a `ValueError` is raised.
By using `raise from`, we raise a new `ValueError` with a custom message "Conversion error: '{s}' is not a valid number", while preserving the context of the original exception.
Thus, in the `try` block of `main`, if a `ValueError` occurs, the error is caught and printed with the additional context.
Conversion error: 'abc' is not a valid number
I hope this guide was helpful! If you have any more questions or want to dive deeper into any aspect, feel free to write in the field below.