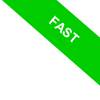
Conditional Control Statement "if-else" in Octave
In this lesson, I will explain how to create an if-else conditional structure in Octave.
What is a conditional structure? It is an instruction that allows you to execute one part of code or another depending on whether a particular condition is met.
In Octave, you can create a conditional structure using the if-elseif-else-endif instruction.
if condition1
code1
else if condition2
code2
else
code3
endif
How does it work?
If the main condition following the "if" statement is true, the program executes the first block of code (code1) and exits the conditional structure.
If the main condition is false, the program checks if the "else if" condition (condition2) is true, and executes the second block of code (code2) if it is, then exits the conditional structure.
Note. You can also include multiple "else if" clauses in a conditional structure, by writing them one after the other.
If none of the conditions are true, the program executes the block of code (code3) after the "else" statement.
Always remember to close the conditional structure with the "endif" statement.
Note. The "else if" and "else" statements are optional. You can also construct a conditional structure without using them.
Here's a practical example.
This script prompts the user to enter a number.
x=input("enter a number ")
if (x==0)
disp("zero")
elseif (x==1)
disp("one")
elseif (x>0)
disp("the number is positive")
else
disp("the number is negative")
endif
After checking the number, proceed to verify if it is equal to zero (if), equal to one (else if), or a positive number (else if).
If none of these conditions hold true, then the number is definitely negative (else).
Example 2
The "else if" conditions are optional. If you don't need them, you can omit them from the conditional structure.
For instance, this conditional structure comprises solely the "if" and "else" statements.
x=input("enter a number ")
if (x==0)
disp("zero")
else
disp("another number")
endif
If the main condition is true (if), it will print the text "zero" to the screen.
On the contrary, if it is false (else), it will print the text "another number".
Example 3
The 'else' clause is optional. If you don't need it, you can omit it.
For instance, this script only checks the main condition.
x=input("enter a number")
if (x==0)
disp("zero")
endif
If the main condition (if) is true, it prints the text 'zero' to the screen.
Otherwise, the script does nothing and exits the conditional structure.