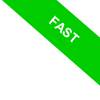
How to program in Python
Do you want to learn how to program in Python? In these tutorials, I will explain how to program in the Python language, starting from scratch.
What is the Python language? It is an open-source programming language characterized by a simple syntax and portability across different operating systems such as Unix, MacOS, and Windows. It belongs to the category of interpreted programming languages, meaning that it does not require compilation. The name "Python" derives from the passion of the language's author, Guido van Rossum, for the British comedy group Monty Python (see the history of the Python language). In this online course, we will be using version Python 3 or higher.
Introduction to the Python language
Python is one of the most versatile programming languages in the world.
Its syntax is clear and readable, making it easy to learn.
It is a cross-platform language, which means that it is available for all major operating systems, including Windows, Linux, and Macintosh.
Python is already included in Linux distributions and Macintosh computers, so it does not need to be installed. However, on Windows PCs, you simply need to install the software that interprets the Python language, which is lightweight and easy to install. I will explain how to do this in the next paragraph.
One of Python's most important features is its portability. Programs written in Python can be executed on different platforms without the need to write separate code for each platform.
This is possible thanks to its architecture, which makes programs written in Python independent of the platform on which they are executed.
It is also a multi-paradigm language, meaning that it can be used in procedural, object-oriented, or functional programming.
However, over time, many versions of the Python language have been released, and not all of them are compatible with each other.
Python versions are divided into major and minor versions.
- Major version
Major versions are the main versions that differ by the first number of the version, known as the major number. They are released after several years. For example, Python 1.0 was released in 1994, version 2.0 was released in 2000, and version 3.0 was released in 2008. Retrocompatibility between major versions is not guaranteed. For instance, a program written with Python 2.0 may not work on Python 3.0. - Minor version
Minor versions are the smaller versions of the Python language. They have the same major number and differ by the number after the dot. For example, within major version 3.0, the minor versions are Python 3.1, 3.2, 3.3, 3.4, etc. They are released more frequently, usually every one or two years. Retrocompatibility between minor versions is guaranteed. Minor versions typically implement new features without modifying the already present ones in the same major version.
Personally, I recommend learning the latest available version of the Python language, which can be found on the official website.
Note. After the minor version number, there may be other numbers separated by another dot, indicating the versions that fix bugs in the language. For example, version 3.5.1 is the first bug-fix release of Python 3.5. There may also be additional codes indicating the addition of new features. The letter "a" indicates alpha sub-release, "b" indicates beta sub-release, and "c" indicates verification from the core development. These letters are followed by other sequential numbers (e.g., versions 3.5.1c4, 3.5.1c5, etc.).
A Practical Example
Here is a simple Python script that illustrates a practical example:
- # Enter two numbers
- num1 = input("Enter the first number: ")
- num2 = input("Enter the second number: ")
- # Convert strings to integers
- num1 = int(num1)
- num2 = int(num2)
- # Calculate the sum
- sum = num1 + num2
- # Print the result
- print("The sum of", num1, "and", num2, "is", sum)
- # Check if the number is negative
- if (sum<0):
- print("The number is negative.")
- else:
- print("The number is greater than or equal to zero.")
The script prompts the user to input two numbers, adds them together, and displays the result. It also checks whether the sum is negative and prints an appropriate message.
Here are some tips for programming in Python:
- Unlike in other programming languages, semicolons are not required at the end of statements in Python. Simply start a new line to begin a new statement.
Note. The semicolon is only necessary if you want to write multiple statements on the same line. However, I advise against it because it makes the code less readable.
- You can add comments to your code by using the hash symbol (#). Place it at the beginning of a line or at the end of a statement.
- When writing nested code blocks, you do not need parentheses in Python. Instead, use indentation to indicate the nested statements. The nested statements should be indented a few spaces to the right of the statement that opens the block. Additionally, the opening statement should end with a colon (:).
How to install Python on a Windows PC
In this first lesson I'll explain how to install the Python interpreter on your Windows computer. The python language is open source. You can download, install and use it for free.
How to use Python's interactive shell
In this lesson I'll explain how to use the Python terminal, what it's for and how it works.
How to write the first program
In this lesson I'll explain how to develop your first program in Python and how to run it.
How to make a variabile
In this tutorial I'll explain how to assign a numeric or alphanumeric value to a variable in python. It's very simple.
What are data types? Once you understand how to use a variable, read the lesson on Python data types.
How to comment the code in Python
In the python script you can also insert comments to make the code more readable.
More Python Online Lessons
- How to take user input
- How to concatenate strings
- How to generate a random number
- How to use IF ELSE statement
- How to get minimum value
- How to find maximum value
- How make a cycle FOR or WHILE