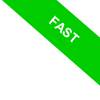
Python's 'from' Keyword
In Python, you have the ability to import certain attributes or functions from a module using the 'from' keyword.
from [module] import [function]
This process enables the use of these imported components directly, without having to prepend the module's name each time.
To elaborate, a module in Python is essentially a library stocked with additional classes, attributes, and functions. You're able to call upon these resources within your Python code. Take the "math" module as an example. It supplements your Python programming with a variety of mathematical functions that aren't found in the language's standard library.
To put this into perspective, let's consider an example.
You wish to import the sqrt() function from the "math" module.
from math import sqrt
Make note that the function name is given without parentheses, hence "sqrt".
This command selectively imports the sqrt() function from the math module, without bringing in the rest of the module's contents.
You're now able to directly utilize the sqrt() function, with no need for a "math" prefix.
print(sqrt(16))
In this scenario, the sqrt() function is calculating the square root of 16, providing a result of
4.0
Similar to the use of the `import` statement, the `from import` statement allows the incorporation of the **"as" clause** to rename an attribute for clarity or simplicity in your code. Take the following snippet, for example: it imports the `sqrt()` function from the math module, assigning it the alias "squareroot" for easier reference:
Similarly to how we utilize the import statement, the "as" clause can be applied in the from import statement to elegantly rename an attribute. This technique streamlines code readability and maintenance.
Consider the example below, where the sqrt() function from the math module is imported under the alias "squareroot", demonstrating a practical application of aliasing:
from math import sqrt as squareroot
y=squareroot(16)
print(y)
4.0
The 'from' keyword can also be employed to import multiple specific functions from a module, distinguished by commas.
For instance, let's import the sqrt() and pow() functions from the "math" module.
from math import sqrt, pow
Following their import, you can utilize these functions directly, bypassing the need for a module name prefix.
print(pow(2,3))
Here, the pow() function calculates the power of 2 to the third, yielding a result of
8.0
Moreover, you have the option to use the asterisk "*" symbol to import all attributes and functions from a module.
from math import *
However, caution is advised with this approach. Using the latter command could lead to potential conflicts with existing local variables and functions within your program that share identical names.
As a rule of thumb, it's typically best to use the 'from' keyword to import only those functions from a module that you actually require.
Alternatively, if you find it necessary to import all functions of a module, it's advisable to use the straightforward import statement.
import math
This statement imports all the functions of the "math" module. To use them, however, you will need to append the module's name as a prefix, like so: math.sqrt().
By doing so, you're able to steer clear of any potential conflicts with functions that are already present in your code.