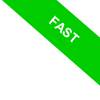
Python's Import Statement
Python's import statement is your gateway to harnessing the power of additional modules in your programs or console.
To use it, simply type:
import module_name
Where "module_name" refers to the module you're planning to bring into memory.
What if you've got several modules to import? No problem! Python allows you to import multiple modules simultaneously, separated by commas.
import module_name1, module_name2
Once you've imported the modules, their functionalities become ready to serve your coding needs.
You might ask, 'What exactly are modules?' Simply put, they're a collection of libraries and packages that extend Python's functionality. The "Math" module, for example, equips Python with a wide array of mathematical functions.
Let's delve into some practical uses of the import statement.
Say you want to import the "math" module.
import math
Once you've done that, the functions within the "math" module are at your fingertips.
print(math.sqrt(16))
This snippet uses the math.sqrt() function to compute the square root of a number.
Note the syntax - the module name "math" followed by a dot and the function name sqrt().
This expression gives you the square root of 16.
4.0
In some cases, you might want to import a module under a different, shorter name. That's where aliases come into play.
Consider this: loading the "numpy" module and assigning it an alias, "np"
import numpy as np
Now you can use np instead of numpy to call the module's functions.
For instance, you can create an array by typing np.array() instead of numpy.array():
a = np.array([1, 2, 3])
These examples bring the entire module into memory.
But Python gives you the flexibility to import specific functions or classes from a module using the "from" keyword.
For instance, to import just the "sqrt" function from the "math" module:
from math import sqrt
Python then imports only the "sqrt" function from "math", bypassing the rest.
print(sqrt(16))
This gives you the square root of 16:
4.0
If you want all functions from a module, use the asterisk "*" with the "from" keyword.
from math import *
This line imports all the functions of the "math" module into memory.
For instance, type sin(0) to calculate the sine of zero.
print(sin(0))
Here, you calculate the sine of zero, getting:
0.0
When using the "from" keyword, remember that you don't need to specify the module name "math" when calling the functions.
For example, to call the sin() function, you just type in the function name sin(), you don't need to write math.sin().
However, this can cause conflicts in your namespace if you've already used the same function names elsewhere in your code.
For instance, if you've defined a sin() function, the imported sin() from "math" would overwrite it.
To avoid such issues, stick to importing only what you need from each module.
Or, import the entire module and access its functions using the module's name. This ensures each function has a unique identifier, like math.sin instead of just sin().