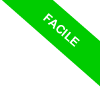
Arithmetic Operators in Java
Arithmetic operators let you perform mathematical calculations in a program, much like you would with a calculator.
In Java, you have operators for addition, subtraction, multiplication, division, and more. These are essential tools for instructing the computer on how to handle numbers.
Here are the key arithmetic operators in Java:
Operator | Description | Example |
---|---|---|
+ | Addition | a + b |
- | Subtraction | a - b |
* | Multiplication | a * b |
/ | Division | a / b |
% | Modulo | a % b |
Let’s start with addition and see how it works.
Addition (`+`)
Imagine you have two numbers and want to add them together—it’s simple, right? Here’s how you do it:
int a = 5;
int b = 3;
int result = a + b;
System.out.println("The result of the addition is: " + result);
8
So, what’s happening here? Java takes the value of `a` (which is 5) and the value of `b` (which is 3), adds them together, and stores the result (which is 8) in the `result` variable. Then, we print it out. Easy enough, right?
Subtraction (`-`)
Now for subtraction. If you want to find the difference between two numbers, it works just like addition, but with the `-` symbol:
int a = 10;
int b = 4;
int difference = a - b;
System.out.println("The result of the subtraction is: " + difference);
6
Here, we subtract 4 from 10, giving us 6. Straightforward.
Multiplication (`*`)
Next, let’s talk about multiplication. This comes in handy when you need to calculate something like the total price of a product multiplied by the quantity:
int price = 7;
int quantity = 5;
int total = price * quantity;
System.out.println("The total is: " + total);
35
Java will take 7 and multiply it by 5, resulting in 35. That’s how you calculate the total cost.
Division (`/`)
Division is a bit more interesting. When you divide two integers, the result will also be an integer. For example:
int dividend = 20;
int divisor = 4;
int divisionResult = dividend / divisor;
System.out.println("The result of the division is: " + divisionResult);
5
In this case, 20 divided by 4 equals 5. So far, so good.
However, if we divide 20 by 3, the result is still an integer:
int dividend = 20;
int divisor = 3;
int divisionResult = dividend / divisor;
System.out.println("The result of the division is: " + divisionResult);
6
Instead of getting 6.6666 (which is the exact result), Java rounds it down to 6 because we’re dealing with integers.
To get a decimal result, you’ll need to use `double` values:
double dividend = 20.0;
double divisor = 3.0;
double divisionResult = dividend / divisor;
System.out.println("The decimal result of the division is: " + divisionResult);
6.6666
Now you get 6.6666, just as expected.
Modulo (`%`)
The modulo operator is interesting because it gives you the remainder of a division. For example, if you divide 10 by 3, you get 3 with a remainder of 1. The modulo operator returns that remainder:
int dividend = 10;
int divisor = 3;
int remainder = dividend % divisor;
System.out.println("The remainder of the division is: " + remainder);
1
Here, the remainder is 1 because 3 goes into 10 three times, leaving a remainder of 1.
This operator is especially useful if you want to check whether a number is even or odd:
int number = 7;
if (number % 2 == 0) {
System.out.println(number + " is even.");
} else {
System.out.println(number + " is odd.");
}
is odd
If `number % 2` equals zero, the number is divisible by 2, so it’s even. Otherwise, it’s odd.
Other Useful Operators
Besides these, there are increment (`++`) and decrement (`--`) operators, which are great for increasing or decreasing a variable by one. Here’s an example of the increment operator:
int a = 5;
a++; // equivalent to a = a + 1;
System.out.println("After increment, a is: " + a);
6
And here’s an example of the decrement operator:
int a = 5;
a--; // equivalent to a = a - 1;
System.out.println("After decrement, a is: " + a);
4
In conclusion, arithmetic operators are fundamental building blocks for any Java program that needs to perform calculations. Even though they might seem simple, they’re powerful tools that can be combined in sophisticated ways to solve a wide range of problems.
The key is understanding how they work at a basic level, and then building on that knowledge. Start with the simple concepts and gradually deepen your understanding.
I hope this gives you a clearer idea of how to use these operators!